(Remove text) Write a program that removes all the occurrences of a specified string from a text file. For example, invoking java Exercise12_11 John filename removes the string John from the specified file. Your program should get the arguments from the command line.并且创建文本文件
时间: 2024-03-08 12:49:39 浏览: 17
以下是一个Java程序示例,可以从文本文件中删除指定字符串:
```java
import java.io.*;
public class RemoveStringFromFile {
public static void main(String[] args) {
if (args.length != 2) {
System.out.println("Usage: java RemoveStringFromFile string filename");
System.exit(1);
}
String stringToRemove = args[0];
String filename = args[1];
// 读取文件内容
StringBuilder sb = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = reader.readLine()) != null) {
sb.append(line).append(System.lineSeparator());
}
} catch (IOException e) {
System.out.println("Error reading file: " + filename);
System.exit(1);
}
// 删除指定字符串
String content = sb.toString().replaceAll(stringToRemove, "");
// 写入文件
try (BufferedWriter writer = new BufferedWriter(new FileWriter(filename))) {
writer.write(content);
} catch (IOException e) {
System.out.println("Error writing file: " + filename);
System.exit(1);
}
System.out.println("String " + stringToRemove + " removed from file " + filename);
}
}
```
该程序从命令行获取要删除的字符串和文件名,读取指定文件的内容,删除所有出现的指定字符串,然后将修改后的内容写回到原始文件中。使用System.lineSeparator()可以跨平台地获取换行符。可以使用以下命令来编译和运行该程序:
```
javac RemoveStringFromFile.java
java RemoveStringFromFile John file.txt
```
该程序会从file.txt文件中删除所有出现的字符串"John"。如果文件不存在或无法读取/写入,程序将终止并输出错误消息。
相关推荐
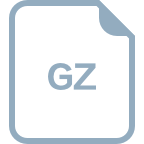
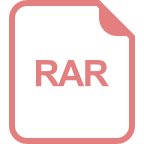
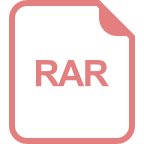
















