Arduino用C语言写一个用中断来控制步进电机带动皮带轮行进的代码
时间: 2024-05-06 12:14:50 浏览: 13
以下是一个简单的示例代码,使用Arduino的中断功能来控制步进电机带动皮带轮行进:
```c
// Step motor control using interrupts
// 控制步进电机,使用中断
// Define step motor pins (IN1, IN2, IN3, IN4)
// 定义步进电机引脚(IN1,IN2,IN3,IN4)
#define IN1 2
#define IN2 3
#define IN3 4
#define IN4 5
// Define interrupt pin for belt wheel sensor
// 定义皮带轮传感器中断引脚
#define SENSOR_PIN 6
// Define distance for each step of the belt wheel
// 定义皮带轮每一步的距离
#define STEP_DISTANCE 10
volatile int step = -1; // Current step of the motor
volatile bool direction = false; // Motor direction (false = forward, true = backward)
volatile int stepsPerRevolution = 200; // Number of steps per revolution of the motor
void setup() {
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
pinMode(SENSOR_PIN, INPUT_PULLUP);
// Attach interrupt to the sensor pin
// 将中断附加到传感器引脚
attachInterrupt(digitalPinToInterrupt(SENSOR_PIN), sensorInterrupt, FALLING);
// Set up the motor
// 设置电机
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
}
void loop() {
// Move the motor one step in the current direction
// 在当前方向上移动电机一步
if (direction == false) {
step++;
if (step >= stepsPerRevolution) {
step = 0;
}
} else {
step--;
if (step < 0) {
step = stepsPerRevolution - 1;
}
}
moveMotor(step % 4);
}
// Interrupt service routine for the sensor pin
// 传感器引脚的中断服务例程
void sensorInterrupt() {
// Change direction when the belt wheel reaches the end
// 当皮带轮到达终点时改变方向
direction = !direction;
}
// Move the motor to the specified step
// 将电机移动到指定的步骤
void moveMotor(int step) {
switch(step) {
case 0:
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
break;
case 1:
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
break;
case 2:
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
break;
case 3:
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
break;
}
delayMicroseconds(1000); // Delay for motor to move
}
```
在这个代码中,我们定义了步进电机的引脚(IN1、IN2、IN3、IN4)、皮带轮传感器的中断引脚(SENSOR_PIN)、每个步骤的距离(STEP_DISTANCE)和每个电机转一圈需要的步数(stepsPerRevolution)。然后我们在setup()函数中设置引脚模式和电机初始化,以及将中断附加到传感器引脚上。在loop()函数中,我们根据当前的方向移动电机,并使用moveMotor()函数控制电机的运动。在sensorInterrupt()函数中,我们检测到传感器的触发信号时,改变电机的方向。
请注意,这只是一个简单的示例代码,实际应用中可能需要更多的控制逻辑和保护措施,以确保系统的可靠性和安全性。
相关推荐
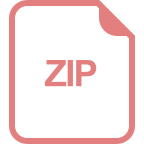
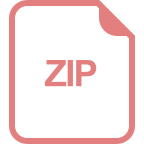














