python定义停用词表和自定义词典
时间: 2023-11-06 21:04:37 浏览: 238
定义停用词表:
在Python中,可以使用列表或集合来定义停用词表。停用词表是指那些在文本处理过程中无需考虑的词语,例如“的”、“了”等等。
以下是使用列表定义停用词表的示例代码:
```python
stop_words = ["的", "了", "和", "是", "就", "都", "而", "及", "与", "或", "等", "也"]
```
以下是使用集合定义停用词表的示例代码:
```python
stop_words = {"的", "了", "和", "是", "就", "都", "而", "及", "与", "或", "等", "也"}
```
定义自定义词典:
在Python中,可以使用字典来定义自定义词典。自定义词典是指那些在文本处理过程中需要特殊处理的词语,例如“人工智能”、“机器学习”等等。
以下是使用字典定义自定义词典的示例代码:
```python
custom_dict = {"人工智能": 100, "机器学习": 90, "深度学习": 80}
```
在这个自定义词典中,每个词语都与一个权重相关联。这个权重可以用于在文本处理过程中进行特殊处理。
相关问题
Python使用jieba进行分词,并应用停用词和自定义的词典
在Python中,jieba是一个常用的中文分词库,它提供了精确、快速的词法分析功能。如果你想要使用jieba进行中文文本的分词,同时移除停用词并应用自定义词典,可以按照以下步骤操作:
首先,确保你已经安装了jieba库,如果没有安装,可以用pip进行安装:
```bash
pip install jieba
```
然后,你可以通过以下步骤来进行分词、去停用词和应用自定义词典:
1. 导入所需的模块:
```python
import jieba
from jieba import posseg as pseg
from collections import Counter
```
2. 加载停用词表:
```python
stop_words_path = 'path_to_your_stopwords.txt'
with open(stop_words_path, 'r', encoding='utf-8') as f:
stop_words = set(line.strip() for line in f)
```
3. 自定义词典(如果有的话),通常是一个dict,键是词语,值是可以忽略或替换的标记:
```python
custom_dict = {'自定义词1': None, '自定义词2': '替换词'}
```
4. 分词并去除停用词:
```python
def process_text(text, custom_dict=custom_dict, stop_words=stop_words):
seg_list = pseg.cut(text)
filtered_words = [(word, flag) for word, flag in seg_list if word not in stop_words and (not custom_dict or word not in custom_dict)]
return [word for word, flag in filtered_words]
text = "这是要进行分词的文本,其中包含一些停用词和自定义词。"
filtered_segments = process_text(text)
```
5. 可能的话,还可以统计词频或做一些进一步的文本分析:
```python
counter = Counter(filtered_segments)
most_common_words = counter.most_common()
```
批量读取文件夹里的txt文件,根据停用词词表以及词表使用最大逆向算法进行分词,词表说明了哪些字的组合可以被算作一个词,停用词表说明哪些没有实意的词可以去掉,其中词表和停用词表均为excel文件,将分词结果以同名文件储存在另一个文件夹里
好的,这里提供一个修改版的代码,用于读取Excel文件中的词表和停用词表,并使用jieba分词库进行分词。
```python
import os
import jieba
import pandas as pd
# 读取词表和停用词表
def read_excel(filename):
df = pd.read_excel(filename, header=None)
words = set(df[0].tolist())
return words
userdict = read_excel('userdict.xlsx')
stopwords = read_excel('stopwords.xlsx')
# 加载自定义词典和停用词表
for word in userdict:
jieba.add_word(word)
stopwords = stopwords.union(set(jieba.get_stop_words()))
# 最大逆向匹配算法
def cut(string):
seg_list = []
max_len = 5 # 词的最大长度
while string:
word = None
for i in range(max_len, 0, -1):
if len(string) >= i and string[-i:] not in stopwords:
word = string[-i:]
seg_list.append(word)
string = string[:-i]
break
if word is None:
seg_list.append(string[-1])
string = string[:-1]
return seg_list[::-1]
# 遍历文件夹,处理每个txt文件
input_folder = 'input_folder'
output_folder = 'output_folder'
for filename in os.listdir(input_folder):
if filename.endswith('.txt'):
# 读取文件内容
with open(os.path.join(input_folder, filename), 'r', encoding='utf-8') as f:
content = f.read().strip()
# 分词
seg_list = jieba.cut(content)
seg_list = [seg.strip() for seg in seg_list if seg.strip() not in stopwords]
# 写入文件
with open(os.path.join(output_folder, filename), 'w', encoding='utf-8') as f:
f.write(' '.join(seg_list))
```
这段代码中使用了pandas库来读取Excel文件中的词表和停用词表。首先定义了一个 `read_excel` 函数,用于读取Excel文件中的内容,并将其转换为集合。然后加载自定义词典和停用词表,使用jieba分词库进行分词并去掉停用词,最后将分词结果写入同名文件中。请注意在使用前将 `input_folder` 和 `output_folder` 改为实际的文件夹路径,并将 `userdict.xlsx` 和 `stopwords.xlsx` 改为实际的词典和停用词表文件名。
阅读全文
相关推荐
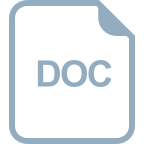
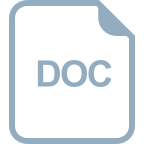
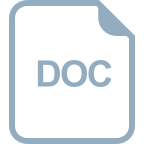
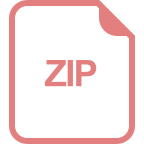



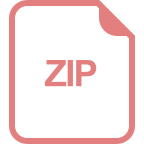
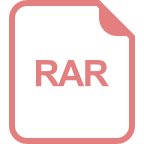
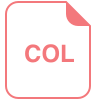





