用C语言实现中缀表达式转化为后缀表达式,写出代码
时间: 2024-05-08 19:19:45 浏览: 17
好的,非常感谢您的提问。以下是使用C语言实现中缀表达式转化为后缀表达式的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAXSIZE 100
char stack[MAXSIZE]; // 定义一个栈
int top = -1; // 栈顶指针
int isDigit(char ch) {
return ch >= '0' && ch <= '9'; // 判断是否为数字
}
int isOperator(char ch) {
return ch == '+' || ch == '-' || ch == '*' || ch == '/';
}
int priority(char ch) { // 定义操作符优先级
if (ch == '*' || ch == '/') {
return 2;
} else if (ch == '+' || ch == '-') {
return 1;
} else {
return 0; // 括号的优先级最高
}
}
void push(char ch) { // 入栈操作
top++;
stack[top] = ch;
}
char pop() { // 出栈操作
char ch = stack[top];
top--;
return ch;
}
void infixToPostfix(char infix[], char postfix[]) { // 中缀表达式转后缀表达式
int length = strlen(infix);
int i, j = 0;
for (i = 0; i < length; i++) {
char ch = infix[i];
if (isDigit(ch)) { // 数字直接输出
postfix[j] = ch;
j++;
} else if (ch == '(') { // 左括号入栈
push(ch);
} else if (ch == ')') { // 右括号出栈
while (top >= 0 && stack[top] != '(') {
postfix[j] = pop();
j++;
}
pop(); // 弹出'('
} else if (isOperator(ch)) { // 操作符需要考虑优先级
while (top >= 0 && priority(ch) <= priority(stack[top])) {
postfix[j] = pop();
j++;
}
push(ch);
}
}
while (top >= 0) { // 将栈中剩余的操作符输出
postfix[j] = pop();
j++;
}
postfix[j] = '\0'; // 结尾添加'\0'
}
int main() {
char infix[MAXSIZE] = "9+(3-1)*3+10/2"; // 中缀表达式
char postfix[MAXSIZE] = ""; // 后缀表达式
infixToPostfix(infix, postfix);
printf("%s", postfix);
return 0;
}
```
以上就是使用C语言实现中缀表达式转化为后缀表达式的代码,希望对您有所帮助。
相关推荐
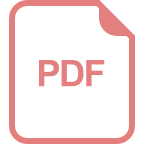














