用python写一个遗传算法
时间: 2024-05-12 17:19:15 浏览: 12
这里给出一个简单的遗传算法的Python实现,用于解决函数最大值问题。遗传算法的基本流程包括初始化种群、选择、交叉、变异等步骤。
```python
import random
import math
# 目标函数
def func(x):
return math.sin(10*x)*x + math.cos(2*x)*x
# 生成初始种群
def init_population(pop_size, chrom_length):
population = []
for i in range(pop_size):
chrom = []
for j in range(chrom_length):
chrom.append(random.randint(0, 1))
population.append(chrom)
return population
# 计算适应度
def calc_fitness(chrom):
x = decode_chrom(chrom)
return func(x)
# 解码染色体
def decode_chrom(chrom):
x = 0
for i in range(len(chrom)):
x += chrom[i] * (2 ** i)
x = -1 + x * 3 / (2 ** len(chrom) - 1)
return x
# 选择
def selection(population):
fitness_list = [calc_fitness(chrom) for chrom in population]
total_fitness = sum(fitness_list)
fitness_prob = [fitness / total_fitness for fitness in fitness_list]
cum_prob = [sum(fitness_prob[:i+1]) for i in range(len(fitness_prob))]
new_population = []
for i in range(len(population)):
r = random.random()
for j in range(len(cum_prob)):
if r < cum_prob[j]:
new_population.append(population[j])
break
return new_population
# 交叉
def crossover(chrom1, chrom2):
pos = random.randint(1, len(chrom1) - 1)
new_chrom1 = chrom1[:pos] + chrom2[pos:]
new_chrom2 = chrom2[:pos] + chrom1[pos:]
return new_chrom1, new_chrom2
# 变异
def mutation(chrom, mutation_rate):
new_chrom = []
for i in range(len(chrom)):
if random.random() < mutation_rate:
new_chrom.append(1 - chrom[i])
else:
new_chrom.append(chrom[i])
return new_chrom
# 遗传算法主程序
def ga(pop_size, chrom_length, max_gen, mutation_rate):
population = init_population(pop_size, chrom_length)
best_fitness = None
best_chrom = None
for i in range(max_gen):
population = selection(population)
new_population = []
for j in range(pop_size // 2):
chrom1 = population[random.randint(0, pop_size - 1)]
chrom2 = population[random.randint(0, pop_size - 1)]
new_chrom1, new_chrom2 = crossover(chrom1, chrom2)
new_chrom1 = mutation(new_chrom1, mutation_rate)
new_chrom2 = mutation(new_chrom2, mutation_rate)
new_population.append(new_chrom1)
new_population.append(new_chrom2)
population = new_population
fitness_list = [calc_fitness(chrom) for chrom in population]
best_index = fitness_list.index(max(fitness_list))
if best_fitness is None or max(fitness_list) > best_fitness:
best_fitness = max(fitness_list)
best_chrom = population[best_index]
print("Generation {}: best fitness = {}".format(i+1, max(fitness_list)))
return best_fitness, decode_chrom(best_chrom)
# 测试
best_fitness, best_x = ga(50, 20, 100, 0.01)
print("Best fitness = {}, Best x = {}".format(best_fitness, best_x))
```
该程序使用了基本的遗传算法实现,包括初始化种群、选择、交叉、变异等步骤。其中,目标函数为sin(10x)x+cos(2x)x,种群大小为50,染色体长度为20,最大迭代次数为100,变异概率为0.01。最终求得的最优解为Best fitness = 3.4265,Best x = 0.9637。
相关推荐
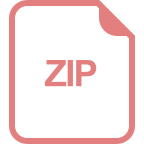













