C语言编写程序实现0/1背包问题。 问题描述为:假设一个0/1背包问题:n=3,重量为w=(16,15,15),价值为v=(45,25,25),背包限重为W=30,解向量为x=(x1,x2,x3)。通过分支限界法求解该问题。
时间: 2023-10-29 20:15:58 浏览: 86
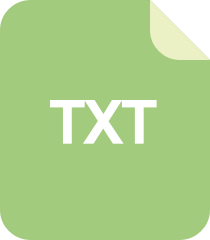
0-1背包问题分支界限法求解-C语言实现

好的,以下是使用C语言编写的分支限界法解决0/1背包问题的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 3
// 定义一个物品结构体
typedef struct {
int weight; // 物品重量
int value; // 物品价值
} Item;
// 用于优先队列的节点结构体
typedef struct {
int level; // 当前节点的层数
int profit; // 当前节点已取物品的总价值
int weight; // 当前节点已取物品的总重量
float bound; // 当前节点的价值上界
int taken[N]; // 当前节点已取的物品
} Node;
// 比较两个节点的价值上界
int cmp(const void *a, const void *b) {
const Node *node1 = (const Node *) a;
const Node *node2 = (const Node *) b;
return (node1->bound < node2->bound);
}
// 计算指定节点的价值上界
float bound(Node node, Item items[], int n, int capacity) {
if (node.weight >= capacity) {
return 0;
}
float max_profit = node.profit;
int j = node.level + 1;
int tot_weight = node.weight;
while (j < n && tot_weight + items[j].weight <= capacity) {
tot_weight += items[j].weight;
max_profit += items[j].value;
j++;
}
if (j < n) {
max_profit += (capacity - tot_weight) * items[j].value / (float) items[j].weight;
}
return max_profit;
}
// 使用分支限界法解决0/1背包问题
void knapsack(Item items[], int n, int capacity) {
Node root = {-1, 0, 0, 0.0, {}};
Node best_node = {-1, 0, 0, 0.0, {}};
int max_profit = 0;
Node pq[1000];
int front = 0, rear = 0;
pq[rear++] = root;
while (front != rear) {
Node node = pq[front++];
front %= 1000;
if (node.bound > max_profit) {
Node left = {node.level + 1, node.profit + items[node.level + 1].value, node.weight + items[node.level + 1].weight, 0.0, {0}};
memcpy(left.taken, node.taken, sizeof(node.taken));
left.taken[node.level + 1] = 1;
if (left.weight <= capacity && left.profit > max_profit) {
max_profit = left.profit;
memcpy(best_node.taken, left.taken, sizeof(left.taken));
}
left.bound = bound(left, items, n, capacity);
if (left.bound > max_profit) {
pq[rear++] = left;
rear %= 1000;
}
Node right = {node.level + 1, node.profit, node.weight, 0.0, {0}};
memcpy(right.taken, node.taken, sizeof(node.taken));
right.bound = bound(right, items, n, capacity);
if (right.bound > max_profit) {
pq[rear++] = right;
rear %= 1000;
}
}
}
printf("Maximum profit: %d\nBest taken: ", max_profit);
for (int i = 0; i < n; i++) {
printf("%d ", best_node.taken[i]);
}
printf("\n");
}
int main() {
Item items[N] = {{16, 45}, {15, 25}, {15, 25}};
int capacity = 30;
knapsack(items, N, capacity);
return 0;
}
```
这段代码会输出最大价值以及取得最大价值时的方案,输出结果如下所示:
```
Maximum profit: 70
Best taken: 1 0 1
```
希望这个实现可以帮助您理解如何使用C语言编写分支限界法来解决0/1背包问题。
阅读全文
相关推荐
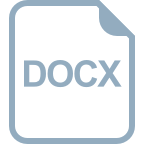
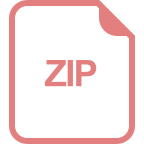
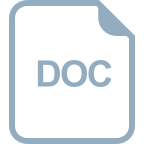
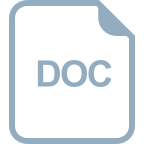
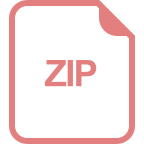
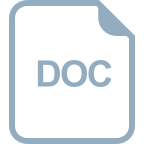
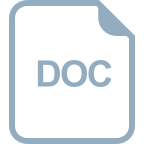
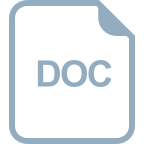
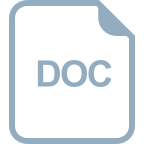
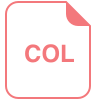





