c语言用伴随矩阵法求矩阵的逆
时间: 2023-06-20 12:07:06 浏览: 238
伴随矩阵法是一种求解矩阵逆的方法,它适用于方阵。假设矩阵A是一个n阶方阵,它的伴随矩阵为Adj(A),则矩阵A的逆矩阵A^-1可以用以下公式求解:
A^-1 = (1/det(A)) * Adj(A)
其中,det(A)为矩阵A的行列式。
以下是用伴随矩阵法求解矩阵逆的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 求解矩阵行列式
float determinant(float **a, int n) {
int i,j,j1,j2;
float det = 0;
float **m = NULL;
if (n < 1) { /* Error */
} else if (n == 1) { /* Shouldn't get used */
det = a[0][0];
} else if (n == 2) {
det = a[0][0] * a[1][1] - a[1][0] * a[0][1];
} else {
det = 0;
for (j1=0;j1<n;j1++) {
m = (float **) malloc((n-1)*sizeof(float *));
for (i=0;i<n-1;i++)
m[i] = (float *) malloc((n-1)*sizeof(float));
for (i=1;i<n;i++) {
j2 = 0;
for (j=0;j<n;j++) {
if (j == j1)
continue;
m[i-1][j2] = a[i][j];
j2++;
}
}
det += pow(-1.0,j1+2.0) * a[0][j1] * determinant(m,n-1);
for (i=0;i<n-1;i++)
free(m[i]);
free(m);
}
}
return(det);
}
// 求解伴随矩阵
void adjoint(float **a, int n, float **adj) {
int i,j,ii,jj,i1,j1;
float det;
float **b;
b = (float **) malloc((n-1)*sizeof(float *));
for (i=0;i<n-1;i++)
b[i] = (float *) malloc((n-1)*sizeof(float));
for (j=0;j<n;j++) {
for (i=0;i<n;i++) {
// Form the adjoint a_ij
i1 = 0;
for (ii=0;ii<n;ii++) {
if (ii == i)
continue;
j1 = 0;
for (jj=0;jj<n;jj++) {
if (jj == j)
continue;
b[i1][j1] = a[ii][jj];
j1++;
}
i1++;
}
// Calculate the determinant
det = determinant(b,n-1);
// Fill in the elements of the adjoint
adj[j][i] = pow(-1.0,i+j+2.0) * det;
}
}
for (i=0;i<n-1;i++)
free(b[i]);
free(b);
}
// 求解矩阵逆
void inverse(float **a, int n, float **inv) {
int i,j;
float det;
float **adj;
adj = (float **) malloc(n*sizeof(float *));
for (i=0;i<n;i++)
adj[i] = (float *) malloc(n*sizeof(float));
// Calculate the adjoint matrix
adjoint(a,n,adj);
// Calculate the determinant
det = 0;
for (i=0;i<n;i++)
det += a[0][i] * adj[0][i];
// Calculate the inverse matrix
for (i=0;i<n;i++) {
for (j=0;j<n;j++)
inv[i][j] = adj[i][j] / det;
}
for (i=0;i<n;i++)
free(adj[i]);
free(adj);
}
int main() {
int i,j,n;
float **a, **inv;
printf("Enter the value of n: ");
scanf("%d",&n);
// Allocate memory for matrices
a = (float **) malloc(n*sizeof(float *));
for (i=0;i<n;i++)
a[i] = (float *) malloc(n*sizeof(float));
inv = (float **) malloc(n*sizeof(float *));
for (i=0;i<n;i++)
inv[i] = (float *) malloc(n*sizeof(float));
// Read in the matrix
printf("Enter the elements of the matrix:\n");
for (i=0;i<n;i++) {
for (j=0;j<n;j++) {
scanf("%f",&a[i][j]);
}
}
// Calculate the inverse matrix
inverse(a,n,inv);
// Display the inverse matrix
printf("The inverse matrix is:\n");
for (i=0;i<n;i++) {
for (j=0;j<n;j++) {
printf("%f ",inv[i][j]);
}
printf("\n");
}
// Free memory
for (i=0;i<n;i++)
free(a[i]);
free(a);
for (i=0;i<n;i++)
free(inv[i]);
free(inv);
return 0;
}
```
阅读全文
相关推荐
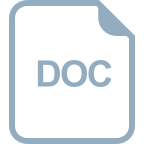
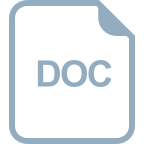
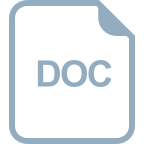


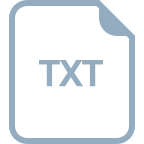
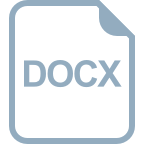
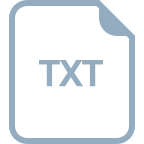
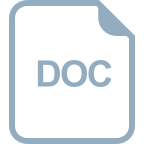









