Collections.sort(a,s)
时间: 2024-02-05 11:07:59 浏览: 31
根据提供的引用内容,我们可以看出这是Java中对数组或集合进行排序的方法。其中,a表示要排序的数组或集合,s表示排序规则。具体来说,s可以是一个实现了Comparator接口的类的对象,也可以是一个Lambda表达式。下面是两个例子:
1. 对数组进行排序
```java
Integer[] arr = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3};
Arrays.sort(arr, (a, b) -> a - b); // 使用Lambda表达式升序排序
System.out.println(Arrays.toString(arr)); // 输出:[1, 1, 2, 3, 3, 4, 5, 5, 6, 9]
```
2. 对集合进行排序
```java
List<String> list = new ArrayList<>();
list.add("apple");
list.add("banana");
list.add("orange");
Collections.sort(list, (a, b) -> b.compareTo(a)); // 使用Lambda表达式降序排序
System.out.println(list); // 输出:[orange, banana, apple]
```
相关问题
Android Studio collections.sort
`Collections.sort()` is a method in Java which sorts a list of objects in ascending order.
In Android Studio, you can use the `Collections.sort()` method to sort an ArrayList of objects. Here's an example:
```java
// Create an ArrayList of strings
ArrayList<String> myStrings = new ArrayList<>();
myStrings.add("banana");
myStrings.add("apple");
myStrings.add("orange");
// Sort the ArrayList in ascending order
Collections.sort(myStrings);
// Print the sorted ArrayList
System.out.println(myStrings);
```
This code will output `[apple, banana, orange]`, which is the sorted version of the original ArrayList.
You can also use the `Collections.sort()` method with custom objects by implementing the `Comparable` interface and overriding the `compareTo()` method. This allows you to define the sorting logic for your objects.
集合List练习 // a.创建商品类,属性:商品编号、商品名称、商品类型、单价、库存、计量单位、产地、品牌 // b.创建List集合类保存多条商品信息 // c.调用Collections.sort()方法,来对集合中的商品进行排序(先按类型升序,如果类型相同再按单价降序)java代码
Sure! Here's an example code that demonstrates the steps you mentioned:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
class Product {
private int productId;
private String productName;
private String productType;
private double price;
private int stock;
private String unit;
private String origin;
private String brand;
// Constructor
public Product(int productId, String productName, String productType, double price, int stock, String unit, String origin, String brand) {
this.productId = productId;
this.productName = productName;
this.productType = productType;
this.price = price;
this.stock = stock;
this.unit = unit;
this.origin = origin;
this.brand = brand;
}
// Getters and Setters
// ...
@Override
public String toString() {
return "Product{" +
"productId=" + productId +
", productName='" + productName + '\'' +
", productType='" + productType + '\'' +
", price=" + price +
", stock=" + stock +
", unit='" + unit + '\'' +
", origin='" + origin + '\'' +
", brand='" + brand + '\'' +
'}';
}
}
public class Main {
public static void main(String[] args) {
// Create a list of products
List<Product> productList = new ArrayList<>();
productList.add(new Product(1, "Product A", "Type B", 10.50, 100, "kg", "USA", "Brand X"));
productList.add(new Product(2, "Product B", "Type A", 5.99, 50, "pcs", "China", "Brand Y"));
productList.add(new Product(3, "Product C", "Type B", 15.75, 200, "kg", "Germany", "Brand Z"));
// Sort the list based on type (ascending) and price (descending)
Collections.sort(productList, new Comparator<Product>() {
@Override
public int compare(Product p1, Product p2) {
int typeComparison = p1.getProductType().compareTo(p2.getProductType());
if (typeComparison == 0) {
return Double.compare(p2.getPrice(), p1.getPrice());
}
return typeComparison;
}
});
// Print the sorted list
for (Product product : productList) {
System.out.println(product);
}
}
}
```
In this example, we create a `Product` class with the specified attributes. We then create a `List<Product>` to store multiple product information. Finally, we use `Collections.sort()` method with a custom `Comparator` to sort the products based on type (ascending) and price (descending).
相关推荐
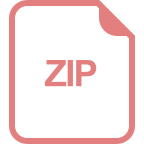
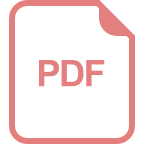
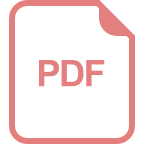












