写一个ceemdan的算法
时间: 2023-11-18 07:11:31 浏览: 84
CEEMDAN(Complete Ensemble Empirical Mode Decomposition with Adaptive Noise)是一种信号分解算法,它是在 EMD(Empirical Mode Decomposition)的基础上发展而来的。下面是一个简单的 CEEMDAN 算法的示例代码:
```python
import numpy as np
from scipy.interpolate import interp1d
from sklearn.neighbors import NearestNeighbors
def eemd(x, max_imf, num_siftings, noise_std):
"""CEEMDAN分解"""
# 初始化
imf = np.zeros((max_imf, len(x)))
x_residue = x.copy()
# 循环分解
for i in range(max_imf):
x_mean = x_residue.mean()
x_std = x_residue.std()
x_noise = np.random.normal(0, noise_std * x_std, len(x_residue))
x_sift = x_residue + x_noise
for j in range(num_siftings):
x_max = x_sift.max()
x_min = x_sift.min()
x_mean = (x_max + x_min) / 2
u = x_sift - x_mean
u1 = u.copy()
imf[i, :] += u
while True:
u_max = u1.max()
u_min = u1.min()
if abs(u_max) < 0.1 * abs(u_min):
break
envelope_up = interp1d([0, len(u1) - 1], [u_max, u_max])(np.arange(len(u1)))
envelope_down = interp1d([0, len(u1) - 1], [u_min, u_min])(np.arange(len(u1)))
m_up = NearestNeighbors(n_neighbors=2).fit(np.concatenate((u1[:, np.newaxis], envelope_up[:, np.newaxis]), axis=1)).kneighbors()[1][:, 1]
m_down = NearestNeighbors(n_neighbors=2).fit(np.concatenate((u1[:, np.newaxis], envelope_down[:, np.newaxis]), axis=1)).kneighbors()[1][:, 1]
mean = (envelope_up + envelope_down) / 2
if u_max > abs(u_min):
mean[m_down] = u1[m_down]
else:
mean[m_up] = u1[m_up]
u2 = u - mean
if (u1 * u2 < 0).sum() > 1:
u1 = u2
else:
break
x_sift -= u
x_residue -= u
if abs(u.mean()) < 0.1 * abs(x_mean):
break
return imf
```
这个函数的输入是一个一维的时间序列信号 x,以及 CEEMDAN 的参数 max_imf(最大的 IMF 分量数)、num_siftings(每个 IMF 分量的迭代次数)和 noise_std(噪声标准差)。输出是一个二维数组 imf,其中每一行是一个 IMF 分量。
阅读全文
相关推荐
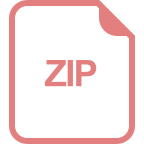
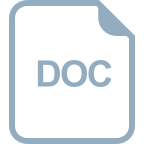
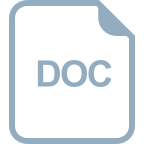











