用c语言编写程序实现顺序表的下列基本操作: (1)初始化顺序表La (2)将La置为空表(3)销毁La(4)在La中插入一个新的元素(5)删除La中的某一元素(6)在La中查找某元素,若找到,则返回它在La中第一次出现的位置,否则返回0 (7)打印输出La中的元素值
时间: 2024-10-15 11:11:57 浏览: 31
在C语言中,我们可以使用结构体和指针来实现顺序表的操作。下面是一个简单的例子,展示了如何完成上述的基本操作:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化顺序表
void initList(Node** La) {
*La = NULL; // 将头指针指向空
}
// 置空表
void clearList(Node** La) {
if (*La != NULL) { // 如果不是空表
Node* temp = *La;
while (temp != NULL) {
Node* oldTemp = temp;
temp = temp->next;
free(oldTemp); // 释放当前节点
}
*La = NULL;
}
}
// 销毁顺序表
void destroyList(Node** La) {
clearList(La);
free(*La); // 释放头指针
}
// 插入新元素
void insert(int value, Node** La) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
newNode->data = value;
newNode->next = *La; // 新节点作为当前表的头部
*La = newNode;
}
// 删除元素
int deleteElement(int target, Node** La) {
if (*La == NULL) return 0; // 判断是否为空表
int pos = 0;
Node* current = *La;
while (current != NULL && current->data != target) {
current = current->next;
pos++;
}
if (current == NULL) return 0; // 没有找到目标元素
Node* temp = current;
current = current->next;
free(temp); // 释放已删除节点的内存
if (current == NULL) *La = NULL; // 如果删除的是最后一个元素,更新头指针
else temp->next = current; // 否则调整相邻节点的连接
return pos; // 返回元素位置
}
// 查找元素并返回索引
int search(int target, Node* La) {
Node* current = La;
for (int i = 0; current != NULL; ++i, current = current->next) {
if (current->data == target) {
return i; // 找到返回其位置
}
}
return 0; // 未找到元素
}
// 打印顺序表
void printList(Node* La) {
if (La == NULL) printf("空表\n");
else {
Node* temp = La;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
}
int main() {
Node* La = NULL;
// 示例用法:初始化、插入、删除、查找和打印
// ...
return 0;
}
```
阅读全文
相关推荐
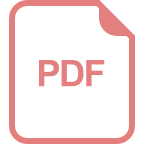
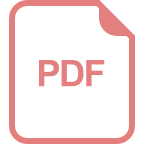
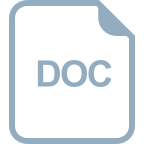
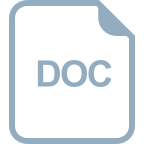
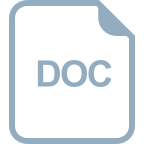
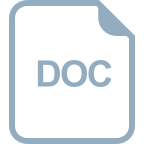
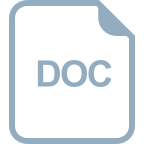
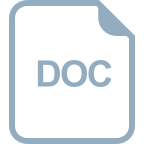
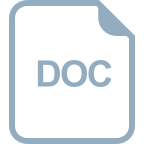
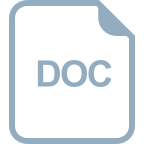
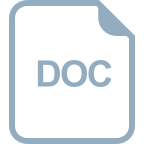
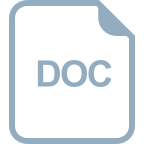






