math类中三维向量
时间: 2023-11-26 11:49:29 浏览: 140
Math类中的三维向量可以使用Vector3类表示。Vector3类用于存储和操作三维空间中的向量,其中包括坐标点的x、y和z分量。您可以使用Vector3类的方法执行向量的加法、减法、标量乘法、点积、叉积等操作。此外,Vector3类还提供了一些有用的属性和方法,例如计算向量的长度、归一化向量等。
相关问题
NET3.5 三维坐标 向量 extension
以下是.NET 3.5中的三维向量扩展方法示例:
```csharp
public static class Vector3Extensions
{
public static Vector3 Cross(this Vector3 vector, Vector3 other)
{
return new Vector3(
vector.Y * other.Z - vector.Z * other.Y,
vector.Z * other.X - vector.X * other.Z,
vector.X * other.Y - vector.Y * other.X);
}
public static float Dot(this Vector3 vector, Vector3 other)
{
return vector.X * other.X + vector.Y * other.Y + vector.Z * other.Z;
}
public static Vector3 Normalize(this Vector3 vector)
{
float length = (float)Math.Sqrt(vector.X * vector.X + vector.Y * vector.Y + vector.Z * vector.Z);
return new Vector3(vector.X / length, vector.Y / length, vector.Z / length);
}
}
```
使用示例:
```csharp
Vector3 v1 = new Vector3(1, 2, 3);
Vector3 v2 = new Vector3(4, 5, 6);
Vector3 crossProduct = v1.Cross(v2);
float dotProduct = v1.Dot(v2);
Vector3 normalizedVector = v1.Normalize();
```
自定义三维向量类,模拟两个三维向量的加减乘除等运算 python
好的,我可以帮你实现一个自定义的三维向量类,并且重载加减乘除等运算符,以下是示例代码:
```python
import math
class Vector3:
def __init__(self, x=0.0, y=0.0, z=0.0):
self.x = x
self.y = y
self.z = z
def __repr__(self):
return f"Vector3({self.x}, {self.y}, {self.z})"
def __add__(self, other):
return Vector3(self.x + other.x, self.y + other.y, self.z + other.z)
def __sub__(self, other):
return Vector3(self.x - other.x, self.y - other.y, self.z - other.z)
def __mul__(self, scalar):
return Vector3(self.x * scalar, self.y * scalar, self.z * scalar)
def __truediv__(self, scalar):
return Vector3(self.x / scalar, self.y / scalar, self.z / scalar)
def dot(self, other):
return self.x * other.x + self.y * other.y + self.z * other.z
def cross(self, other):
return Vector3(self.y * other.z - self.z * other.y,
self.z * other.x - self.x * other.z,
self.x * other.y - self.y * other.x)
def magnitude(self):
return math.sqrt(self.x ** 2 + self.y ** 2 + self.z ** 2)
def normalize(self):
mag = self.magnitude()
if mag > 0:
self.x /= mag
self.y /= mag
self.z /= mag
# 测试代码
v1 = Vector3(1, 2, 3)
v2 = Vector3(4, 5, 6)
print(v1 + v2) # Vector3(5, 7, 9)
print(v1 - v2) # Vector3(-3, -3, -3)
print(v1 * 2) # Vector3(2, 4, 6)
print(v2 / 2) # Vector3(2.0, 2.5, 3.0)
print(v1.dot(v2)) # 32
print(v1.cross(v2)) # Vector3(-3, 6, -3)
v3 = Vector3(3, 0, 0)
v3.normalize()
print(v3) # Vector3(1.0, 0.0, 0.0)
```
这是一个简单的示例,你可以根据需要添加更多的操作符重载和方法。
阅读全文
相关推荐
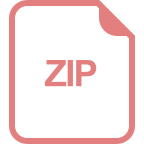
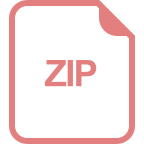
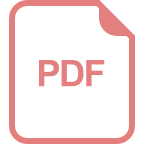














