output_3D[:, :, 0, :] = 0
时间: 2024-05-24 09:12:34 浏览: 99
This code sets all the values in the first channel (third dimension) of a four-dimensional numpy array called output_3D to 0. The syntax [:, :, 0, :] means to select all values in the first and second dimensions (rows and columns), set the third dimension index to 0, and select all values in the fourth dimension.
So if the shape of output_3D is (4, 4, 3, 2), this code would set all the values in the first channel to 0, leaving the other two channels unchanged.
相关问题
ros::init(argc, argv, "kitti_helper"); ros::NodeHandle n("~"); std::string dataset_folder, sequence_number, output_bag_file; n.getParam("dataset_folder", dataset_folder); n.getParam("sequence_number", sequence_number); std::cout << "Reading sequence " << sequence_number << " from " << dataset_folder << '\n'; bool to_bag; n.getParam("to_bag", to_bag); if (to_bag) n.getParam("output_bag_file", output_bag_file); int publish_delay; n.getParam("publish_delay", publish_delay); publish_delay = publish_delay <= 0 ? 1 : publish_delay; ros::Publisher pub_laser_cloud = n.advertise<sensor_msgs::PointCloud2>("/velodyne_points", 2); image_transport::ImageTransport it(n); image_transport::Publisher pub_image_left = it.advertise("/image_left", 2); image_transport::Publisher pub_image_right = it.advertise("/image_right", 2); ros::Publisher pubOdomGT = n.advertise<nav_msgs::Odometry> ("/odometry_gt", 5); nav_msgs::Odometry odomGT; odomGT.header.frame_id = "/camera_init"; odomGT.child_frame_id = "/ground_truth"; ros::Publisher pubPathGT = n.advertise<nav_msgs::Path> ("/path_gt", 5); nav_msgs::Path pathGT; pathGT.header.frame_id = "/camera_init"; std::string timestamp_path = "sequences/" + sequence_number + "/times.txt"; std::ifstream timestamp_file(dataset_folder + timestamp_path, std::ifstream::in); std::string ground_truth_path = "results/" + sequence_number + ".txt"; std::ifstream ground_truth_file(dataset_folder + ground_truth_path, std::ifstream::in); rosbag::Bag bag_out; if (to_bag) bag_out.open(output_bag_file, rosbag::bagmode::Write); Eigen::Matrix3d R_transform; R_transform << 0, 0, 1, -1, 0, 0, 0, -1, 0; Eigen::Quaterniond q_transform(R_transform); std::string line; std::size_t line_num = 0; ros::Rate r(10.0 / publish_delay); 解释一下
这段代码是一个 ROS 节点程序的主要部分,它首先对 ROS 进行初始化,然后获取节点参数,包括数据集文件夹路径、序列号、是否需要将数据保存到 rosbag 文件中等。接着,它创建了一个点云发布器、两个图像发布器、一个里程计发布器和一个路径发布器。这些发布器用于将数据发布到 ROS 系统中。在发布器创建后,该程序读取数据集中的时间戳和地面真实数据文件,并打开 rosbag 文件(如果需要)。接下来,程序进行了一些矩阵变换,然后进入一个循环中,按照一定的频率(10Hz / 发布延迟)发布数据。
读取输出数据 # 读取train.hdf5文件中的二维数组 with h5py.File('train001.hdf5', 'r') as f: data01 = f['increment_4/phase/alpha-Ti/mechanical/O'][:] data02 = f['/increment_4/phase/alpha-Ti/mechanical/epsilon_V^0.0(F)_vM'][:] data02 = data02[:, np.newaxis] data03 = f['/increment_4/phase/alpha-Ti/mechanical/sigma_vM'][:] data03 = data03[:, np.newaxis] data03 = data03 / 1e6 # 归一化到-1和1之间 data03 = (data03 - np.max(data03) / 2) / (np.max(data03) / 2) # 按行连接数组 arr = np.hstack((data01, data02, data03)) # 每1024行提取出来作为一个二维数组 output_arr_reshaped = arr.reshape((-1, 1024, 6)) # 将每个二维数组转换成三维数组 output_arr_3d = [] for i in range(output_arr_reshaped.shape[0]): output_arr_3d.append(np.reshape(output_arr_reshaped[i], (32, 32, 6))) # 将每个三维数组转换成6 * 32 * 32的三维数组 output_arr_6_32_32 = [] for i in range(len(output_arr_3d)): output_arr_6_32_32.append(np.transpose(output_arr_3d[i], (2, 0, 1))) # 对每个5 * 32 * 32的数组进行修改 modified_output_arr = [] for i in range(len(output_arr_6_32_32)): output_arr_i = output_arr_6_32_32[i] output_arr_i = np.array(output_arr_i) output_arr_i = torch.from_numpy(output_arr_i).float() output_arr_i = output_arr_i.unsqueeze(0) modified_output_arr.append(output_arr_i) # 输出第一个三维数组中的第一个纵向二维数组 output_arr01 = modified_output_arr[0] 帮我优化这段代码,使得这段代码功能完全不变的情况下更加精简,并帮我标好每一段的功能
以下是代码的优化和注释:
```python
import h5py
import numpy as np
import torch
# 读取train.hdf5文件中的二维数组
with h5py.File('train001.hdf5', 'r') as f:
# 读取三个二维数组
data01 = f['increment_4/phase/alpha-Ti/mechanical/O'][:]
data02 = f['/increment_4/phase/alpha-Ti/mechanical/epsilon_V^0.0(F)_vM'][:, np.newaxis]
data03 = f['/increment_4/phase/alpha-Ti/mechanical/sigma_vM'][:, np.newaxis]
# 归一化到-1和1之间
data03 = (data03 / 1e6 - np.max(data03) / 2) / (np.max(data03) / 2)
# 按行连接数组
arr = np.hstack((data01, data02, data03))
# 将每1024行提取出来作为一个二维数组
output_arr_reshaped = arr.reshape((-1, 1024, 6))
# 将每个二维数组转换成6 * 32 * 32的三维数组
output_arr_6_32_32 = np.transpose(output_arr_reshaped.reshape((-1, 32, 32, 6)), (3, 1, 2))
# 对每个5 * 32 * 32的数组进行修改
modified_output_arr = [torch.from_numpy(output_arr_6_32_32[i]).float().unsqueeze(0) for i in range(len(output_arr_6_32_32))]
# 输出第一个三维数组中的第一个纵向二维数组
output_arr01 = modified_output_arr[0]
```
注释中标注了每一段代码的功能。代码的优化主要包括:
1. 将读取的三个二维数组合并为一个二维数组时,直接使用 `np.hstack()` 函数即可,无需使用多行代码实现。
2. 将每个二维数组转换成6 * 32 * 32的三维数组时,可以直接使用 `output_arr_reshaped.reshape((-1, 32, 32, 6))` 实现。同时,使用 `np.transpose()` 函数将通道维移动到第一维,代码更加简洁。
3. 在对每个5 * 32 * 32的数组进行修改时,可以使用列表推导式实现,代码更加简洁。同时,可以直接在一行代码中完成 `torch.from_numpy()` 和 `float()` 的转换。
阅读全文
相关推荐
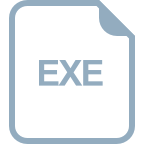
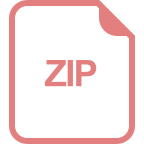














