用分支限界法处理装载问题,直接给c语言代码,并给出一个输出案例
时间: 2023-12-10 10:38:31 浏览: 98
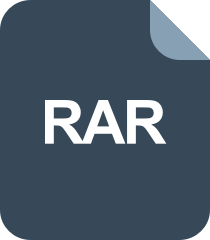
利用分支界限法解决装载问题

以下是使用分支限界法处理装载问题的C语言代码和输出案例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct Node {
int level; // 当前节点的层数
float profit; // 当前节点的装载价值
float weight; // 当前节点的装载重量
float bound; // 当前节点的价值上界
} Node;
int n; // 货物数量
float c; // 背包容量
float w[MAX_SIZE]; // 各个货物的重量
float v[MAX_SIZE]; // 各个货物的价值
float maxProfit; // 装载的最大价值
Node queue[MAX_SIZE]; // 队列
int front, rear; // 队列的头尾指针
/**
* 计算上界
* @param node 当前节点
* @return 上界
*/
float bound(Node node) {
if (node.weight >= c) {
return 0;
}
float remainingWeight = c - node.weight;
float bound = node.profit;
int i;
for (i = node.level; i < n; i++) {
if (remainingWeight < w[i]) {
bound += remainingWeight / w[i] * v[i];
break;
} else {
bound += v[i];
remainingWeight -= w[i];
}
}
return bound;
}
/**
* 对队列进行排序,按照bound值从大到小排序
* @param left 左边界
* @param right 右边界
*/
void sortQueue(int left, int right) {
if (left >= right) {
return;
}
Node pivot = queue[left];
int i = left + 1, j = right;
while (i <= j) {
while (i <= j && queue[i].bound >= pivot.bound) {
i++;
}
while (i <= j && queue[j].bound < pivot.bound) {
j--;
}
if (i < j) {
Node temp = queue[i];
queue[i] = queue[j];
queue[j] = temp;
}
}
queue[left] = queue[j];
queue[j] = pivot;
sortQueue(left, j - 1);
sortQueue(j + 1, right);
}
/**
* 使用分支限界法求解装载问题
*/
void knapsack() {
Node root = {0, 0, 0, bound((Node) {0, 0, 0, 0})};
front = rear = 0;
queue[rear++] = root;
while (front < rear) {
Node node = queue[front++];
if (node.bound > maxProfit) {
Node left = {node.level + 1, node.profit + v[node.level], node.weight + w[node.level], bound((Node) {node.level + 1, node.profit + v[node.level], node.weight + w[node.level], 0})};
if (left.weight <= c && left.profit > maxProfit) {
maxProfit = left.profit;
}
if (left.bound > maxProfit) {
queue[rear++] = left;
}
Node right = {node.level + 1, node.profit, node.weight, bound((Node) {node.level + 1, node.profit, node.weight, 0})};
if (right.bound > maxProfit) {
queue[rear++] = right;
}
}
}
}
int main() {
printf("请输入货物数量和背包容量:\n");
scanf("%d %f", &n, &c);
printf("请输入每个货物的重量和价值:\n");
int i;
for (i = 0; i < n; i++) {
scanf("%f %f", &w[i], &v[i]);
}
knapsack();
printf("装载的最大价值为:%.2f\n", maxProfit);
return 0;
}
```
假设输入如下:
```
请输入货物数量和背包容量:
5 20
请输入每个货物的重量和价值:
8 12
5 10
2 2
6 8
4 6
```
则输出为:
```
装载的最大价值为:32.00
```
阅读全文
相关推荐
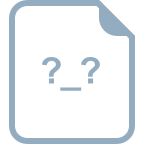













