Write a C language program that reads an expression in a line as input and prints out the result. Only integers and operators below are allowed in the expression: + - * / % ( ) 输入格式: A line of expression. 输出格式: The result.要求能计算连续乘除和括号。
时间: 2024-02-07 12:03:41 浏览: 111
以下是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
int main() {
char expr[100];
int stack[100];
int top = -1;
int i, num1, num2, ans;
printf("请输入表达式:");
fgets(expr, 100, stdin);
for (i = 0; expr[i] != '\n'; i++) {
if (expr[i] >= '0' && expr[i] <= '9') {
int num = 0;
while (expr[i] >= '0' && expr[i] <= '9') {
num = num * 10 + (expr[i] - '0');
i++;
}
i--;
stack[++top] = num;
} else if (expr[i] == '(') {
stack[++top] = expr[i];
} else if (expr[i] == ')') {
while (stack[top] != '(') {
num2 = stack[top--];
char op = stack[top--];
num1 = stack[top--];
switch (op) {
case '+':
ans = num1 + num2;
break;
case '-':
ans = num1 - num2;
break;
case '*':
ans = num1 * num2;
break;
case '/':
ans = num1 / num2;
break;
case '%':
ans = num1 % num2;
break;
default:
printf("非法运算符\n");
exit(1);
}
stack[++top] = ans;
}
top--;
} else if (expr[i] == '+' || expr[i] == '-' || expr[i] == '*' || expr[i] == '/' || expr[i] == '%') {
stack[++top] = expr[i];
} else {
printf("非法字符\n");
exit(1);
}
}
while (top > 0) {
num2 = stack[top--];
char op = stack[top--];
num1 = stack[top--];
switch (op) {
case '+':
ans = num1 + num2;
break;
case '-':
ans = num1 - num2;
break;
case '*':
ans = num1 * num2;
break;
case '/':
ans = num1 / num2;
break;
case '%':
ans = num1 % num2;
break;
default:
printf("非法运算符\n");
exit(1);
}
stack[++top] = ans;
}
printf("结果为:%d\n", stack[top]);
return 0;
}
```
该程序首先从标准输入中读取一个表达式,然后使用堆栈来计算表达式的值。堆栈中保存了操作数和运算符。如果遇到一个数字,则将其推入堆栈;如果遇到一个左括号,则将其推入堆栈;如果遇到一个右括号,则将堆栈中的操作数和运算符弹出,计算结果并将其推入堆栈;如果遇到一个运算符,则将其推入堆栈。
在计算表达式时,程序首先弹出堆栈中的操作数和运算符,并根据运算符进行计算,然后将结果推入堆栈。最终,堆栈中仅剩一个元素,即表达式的值。
阅读全文
相关推荐














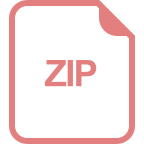
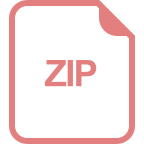