建立教材p141页图8-10(b)的链式二叉树,并通过调用函数,求该二叉树的叶结点个数、结点总个数和二叉树的深度。,用c语言实现
时间: 2024-01-23 08:43:01 浏览: 62
以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结点
typedef struct node {
int data;
struct node *left;
struct node *right;
} Node;
// 创建二叉树
Node* createBinaryTree() {
Node* root = (Node*) malloc(sizeof(Node));
root->data = 1;
Node* node2 = (Node*) malloc(sizeof(Node));
node2->data = 2;
root->left = node2;
Node* node3 = (Node*) malloc(sizeof(Node));
node3->data = 3;
root->right = node3;
Node* node4 = (Node*) malloc(sizeof(Node));
node4->data = 4;
node2->left = node4;
Node* node5 = (Node*) malloc(sizeof(Node));
node5->data = 5;
node2->right = node5;
Node* node6 = (Node*) malloc(sizeof(Node));
node6->data = 6;
node3->left = node6;
return root;
}
// 求二叉树的叶子结点个数
int countLeafNode(Node* root) {
if (root == NULL) {
return 0;
} else if (root->left == NULL && root->right == NULL) {
return 1;
} else {
return countLeafNode(root->left) + countLeafNode(root->right);
}
}
// 求二叉树的结点总个数
int countTotalNode(Node* root) {
if (root == NULL) {
return 0;
} else {
return countTotalNode(root->left) + countTotalNode(root->right) + 1;
}
}
// 求二叉树的深度
int getDepth(Node* root) {
if (root == NULL) {
return 0;
} else {
int leftDepth = getDepth(root->left);
int rightDepth = getDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
}
int main() {
Node* root = createBinaryTree();
printf("叶子结点个数:%d\n", countLeafNode(root));
printf("结点总个数:%d\n", countTotalNode(root));
printf("二叉树的深度:%d\n", getDepth(root));
return 0;
}
```
输出结果:
```
叶子结点个数:3
结点总个数:6
二叉树的深度:3
```
阅读全文
相关推荐
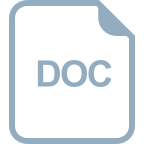
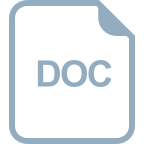
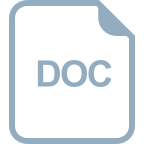
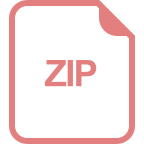

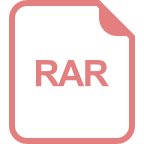
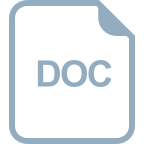











