ue5 c++ if
时间: 2024-08-28 17:00:53 浏览: 90
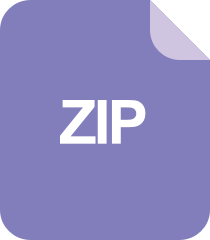
UE4 C++JSON读取示例.zip
在Unreal Engine (UE) 5的C++中,`if`语句用于条件控制流程。它允许程序根据某个条件是否满足来执行不同的代码块。基本的`if`语句的语法类似于这样:
```cpp
if (condition)
{
// 如果 condition 为真,执行这里的代码
}
```
你也可以使用`else`来指定条件不满足时的代码:
```cpp
if (condition)
{
// 条件为真的代码
}
else
{
// 条件为假的代码
}
```
还有三元运算符 `?:`,可以简化单分支`if...else`结构:
```cpp
result = condition ? true_value : false_value;
```
在这个表达式中,如果`condition`为真,则`true_value`会被赋值给`result`;否则`false_value`会被赋值。
阅读全文
相关推荐
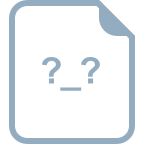
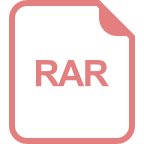















