已知平面内两点和一个点集,用两点形成的直线把点集进行分类,用python实现
时间: 2023-07-16 09:15:08 浏览: 84
我们可以先定义一个函数,输入为两个点和点集,输出为两个分类后的点集。
```python
def classify_points_by_line(point1, point2, points):
"""
Classify a set of points into two groups based on a line defined by two points.
Args:
point1: tuple, the first point
point2: tuple, the second point
points: list of tuples, the set of points to be classified
Returns:
tuple of two lists, the two classified sets of points
"""
# Initialize two empty lists to store points on each side of the line.
group1 = []
group2 = []
# Calculate the slope and y-intercept of the line.
if point1[0] == point2[0]:
slope = None
intercept = point1[0]
else:
slope = (point2[1] - point1[1]) / (point2[0] - point1[0])
intercept = point1[1] - slope * point1[0]
# Classify each point based on its position relative to the line.
for point in points:
if slope is None:
x = intercept
if point[0] < x:
group1.append(point)
elif point[0] > x:
group2.append(point)
else:
y = slope * point[0] + intercept
if point[1] < y:
group1.append(point)
elif point[1] > y:
group2.append(point)
# Return the two groups of points.
return group1, group2
```
这个函数中,我们首先初始化两个空列表来存储分别在直线的两边的点。然后,我们计算出直线的斜率和 y 截距。如果直线竖直,斜率为 None,此时直线的方程只有一个变量 x,我们可以用 x = intercept 来计算点在直线上的位置。如果直线不竖直,我们可以用 y = slope * x + intercept 来计算点在直线上的位置。最后,我们根据每个点在直线的哪一边将其分类到 group1 或 group2 中,并返回这两个列表。
下面是一个简单的例子:
```python
point1 = (0, 0)
point2 = (1, 1)
points = [(0.5, 0.5), (0.8, 0.8), (0.2, 0.2), (1.5, 1.5)]
group1, group2 = classify_points_by_line(point1, point2, points)
print("Group 1:", group1)
print("Group 2:", group2)
```
输出:
```
Group 1: [(0.5, 0.5), (0.2, 0.2)]
Group 2: [(0.8, 0.8), (1.5, 1.5)]
```
在这个例子中,我们用 (0, 0) 和 (1, 1) 这两个点定义了一条直线,然后将点集 [(0.5, 0.5), (0.8, 0.8), (0.2, 0.2), (1.5, 1.5)] 按照这条直线分成了两组。其中,(0.5, 0.5) 和 (0.2, 0.2) 在直线的左边,被分为 group1,(0.8, 0.8) 和 (1.5, 1.5) 在直线的右边,被分为 group2。
阅读全文
相关推荐
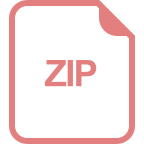
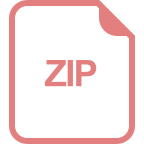











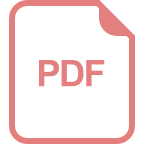
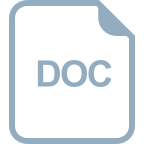
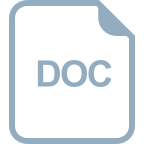
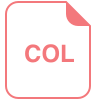

