def fitness(individual): predicted = sum([individual[kk] * nums1[kk][:] for kk in range(gene_length)]) / gene_length mse = np.mean((predicted - target) ** 2) # 均方根误差 correlation = np.corrcoef(predicted, target)[0, 1] # 相关系数 fitness_score = correlation / (mse + 1)# 适应度函数 return fitness_score在这个适应函数中我还想让nums之和为1
时间: 2023-06-16 09:04:08 浏览: 52
你可以在适应度函数中添加一个惩罚项,使得所有nums的和为1。例如,你可以计算所有nums的和与1之间的差值,然后将其加到适应度分数中。具体实现如下:
```
def fitness(individual):
nums_sum = sum(individual)
if nums_sum != 1: # 惩罚项
penalty = abs(nums_sum - 1)
else:
penalty = 0
predicted = sum([individual[kk] * nums1[kk][:] for kk in range(gene_length)]) / gene_length
mse = np.mean((predicted - target) ** 2)
correlation = np.corrcoef(predicted, target)[0, 1]
fitness_score = correlation / (mse + 1) - penalty # 加上惩罚项
return fitness_score
```
这样,如果所有nums的和不为1,适应度分数就会被减去一个惩罚项,从而降低该个体的适应度分数。
相关问题
def fitness(individual): predicted = sum([individual[kk] * nums1[kk] for kk in range(0,gene_length,1)]) / gene_length mse = np.mean((predicted - target) ** 2) # 均方根误差 correlation = np.corrcoef(predicted, target)[0, 1] # 相关系数 #total_sum = np.sum(individual) #penalty = abs(total_sum - 1) # 总和与1的差作为惩罚项 fitness_score = correlation / (mse + 1) # 适应度函数 return fitness_score #给予每个模式在格点上的权重 def init_population(pop_size, gene_length): population = [] for i in range(pop_size): individual = [random.uniform(0, 16) for bb in range(gene_length)] total_sum = sum(individual) scaling_factor = total_sum / 1.0 individual = [x / scaling_factor for x in individual] population.append(individual) return population 这段代码中加一个条件就是individual之和应该是等于gene_length
很抱歉,我之前的回答有误。如果你想要individual的元素之和等于gene_length,可以按照如下方式修改代码:
```
def init_population(pop_size, gene_length):
population = []
for i in range(pop_size):
individual = [random.uniform(0, 16) for bb in range(gene_length)]
total_sum = sum(individual)
scaling_factor = gene_length / total_sum
individual = [x * scaling_factor for x in individual]
population.append(individual)
return population
```
这里的scaling_factor是总和与gene_length的比例,将它乘以individual中的每个元素,可以使得它们的和等于gene_length。
def test(): correct = 0 total = 0 with torch.no_grad: for data in test_loader: x, y =data y_pred = model(x) _, predicted = torch.max(y_pred, dim=1) total += y.size(0) correct += (predicted == y).sum().item() print('accuracy on test_data:%d %%' % (100 *correct/total))
这段代码存在一个语法错误,在第 4 行应该调用 `torch.no_grad()` 方法而不是 `torch.no_grad` 属性。`torch.no_grad()` 方法是一个上下文管理器,它使得在该上下文中计算的所有张量都不会被跟踪梯度,从而提高了计算效率。
正确的代码应该是:
```
def test():
correct = 0
total = 0
with torch.no_grad():
for data in test_loader:
x, y = data
y_pred = model(x)
_, predicted = torch.max(y_pred, dim=1)
total += y.size(0)
correct += (predicted == y).sum().item()
print('accuracy on test_data:%d %%' % (100 * correct/total))
```
注意,在 `with` 语句中调用 `torch.no_grad()` 方法之后,所有在该上下文中计算的张量都不会被跟踪梯度,这有助于提高计算效率。然而,如果需要计算梯度,则需要退出该上下文并调用 `backward()` 方法。
相关推荐
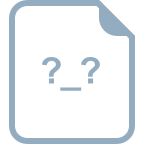
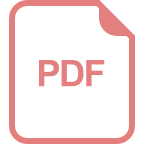
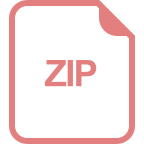












