v-for怎么控制起点与终点
时间: 2024-09-26 12:01:52 浏览: 45
`v-for` 是 Vue.js 中用于迭代数组或对象的一个指令,它可以根据给定的数据范围生成 HTML 元素。如果你想要控制 `v-for` 的起始索引和结束索引,你可以通过两个额外的属性来实现:
1. **`:start`** 或 **`:from`**: 设置循环的开始索引。例如:
```html
<ul>
<li v-for="item in items" :start="startIndex"></li>
</ul>
```
2. **`:to`** 或 **`:end`**: 设置循环的结束索引,这将排除指定的元素。例如:
```html
<ul>
<li v-for="(item, index) in items.slice(startIndex, endIndex)" :key="index"></li>
</ul>
```
这里 `items.slice(startIndex, endIndex)` 会截取从 `startIndex` 到(`endIndex - 1`)的元素。
如果你想动态地改变起点和终点,可以使用 `v-bind` 绑定变量到这些属性,然后在 JavaScript 中根据需要更新那些变量。
相关问题
用c语言写 TODO:找到两个点之间的所有路径 功能描述:从起点位置出发深度优先遍历图g中能访问的各个顶点,找到指定起点到指定终点的路径信息 调用PrintVInfo(graph *g)方法,打印出路径中的顶点信息。 参数说明:g-graph型指针参数 startV-int型起点位置 endV-终点的位置 返回值说明:无
好的,这是一个比较典型的深度优先搜索问题,可以使用递归的方式来实现。下面是一个可能的现,供您参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_VERTICES 100
// 图的邻接表表示
typedef struct node {
int vertex;
struct node *next;
} node;
typedef struct graph {
node *adjList[MAX_VERTICES];
int visited[MAX_VERTICES];
int verticesCount;
} graph;
// 创建新的节点
node *createNode(int v) {
node *newNode = malloc(sizeof(node));
newNode->vertex = v;
newNode->next = NULL;
return newNode;
}
// 创建新的图
graph *createGraph(int verticesCount) {
graph *g = malloc(sizeof(graph));
g->verticesCount = verticesCount;
for (int i = 0; i < verticesCount; i++) {
g->adjList[i] = NULL;
g->visited[i] = 0;
}
return g;
}
// 添加边
void addEdge(graph *g, int src, int dest) {
node *newNode = createNode(dest);
newNode->next = g->adjList[src];
g->adjList[src] = newNode;
}
// 打印路径信息
void printVInfo(graph *g, int startV, int endV, int *path, int pathLen) {
printf("Path found: ");
for (int i = 0; i < pathLen; i++) {
printf("%d ", path[i]);
}
printf("%d\n", endV);
}
// 深度优先搜索递归实现
void dfs(graph *g, int currentV, int endV, int *path, int pathLen) {
// 把当前节点加入路径
path[pathLen] = currentV;
pathLen++;
// 如果找到终点,打印路径
if (currentV == endV) {
printVInfo(g, path[0], endV, path, pathLen);
return;
}
// 标记当前节点已访问
g->visited[currentV] = 1;
// 遍历当前节点的邻居
node *adjList = g->adjList[currentV];
while (adjList != NULL) {
int adjVertex = adjList->vertex;
if (!g->visited[adjVertex]) {
dfs(g, adjVertex, endV, path, pathLen);
}
adjList = adjList->next;
}
// 标记当前节点已访问结束
g->visited[currentV] = 0;
}
// 找到两个点之间的所有路径
void findAllPaths(graph *g, int startV, int endV) {
int path[MAX_VERTICES];
int pathLen = 0;
dfs(g, startV, endV, path, pathLen);
}
// 测试代码
int main() {
int verticesCount = 6;
graph *g = createGraph(verticesCount);
addEdge(g, 0, 1);
addEdge(g, 0, 2);
addEdge(g, 1, 3);
addEdge(g, 2, 3);
addEdge(g, 3, 4);
addEdge(g, 3, 5);
findAllPaths(g, 0, 4);
return 0;
}
```
这段代码实现了一个简单的无向图(图的结构可以通过 `addEdge` 函数进行修改),并通过调用 `findAllPaths` 函数来找到从起点到终点的所有路径。
需要注意的是,这段代码并没有进行错误处理和内存释放,您可以自行进行修改。同时,这段代码可能并不是最优的实现方式,您可以根据实际情况进行修改和优化。
改正这段代码的错误 LGraph BuildGraph(){ LGraph Graph; Edge E; Vertex V; int Nv,i; printf("输入顶点个数:"); scanf("%d",&Nv); Graph=CreateGraph(Nv); printf("输入边数"); scanf("%d",&(Graph->Ne)); if(Graph->Ne!=0){ E=(Edge)malloc(sizeof(struct ENode)); printf("输入边,格式为:起点小标 终点下标 权重,()"); for(i=0;i<Graph->Ne;i++){ scanf("%d %d %d",&E->V1;&E->V2;E->Weight); InsertEdge(Graph,E); } } printf("输入顶点名称"); for(V=0;V=Graph->Nv;V++){ scanf("%c",&(Graph->G[V].Data); } return Graph; } /*05 深度优先遍历操作 函数名:DFS 说明: 入参:(LGraph Graph,Vertex V) 出参: LGraph Graph 测试输入:0 3 1 0 1 1 1 3 1 1 2 1 A B C D */ void DFS(LGraph Graph,Vertex V){ /*以V为出发点对邻接表存储的图Graph进行DFS搜索*/ PtrToAdjVNode W; Visit(Graph,V); Visited[V]=false; for(W=Graph->G[V].FirstEdge;W=W->Next){ if(!Visited[W->AdjV]){ DFS(Graph,W->AdjV); } } }
以下是改正后的代码:
```
LGraph BuildGraph(){
LGraph Graph;
Edge E;
Vertex V;
int Nv,i;
printf("输入顶点个数:");
scanf("%d", &Nv);
Graph = CreateGraph(Nv);
printf("输入边数:");
scanf("%d", &(Graph->Ne));
if(Graph->Ne != 0){
E = (Edge)malloc(sizeof(struct ENode));
printf("输入边,格式为:起点下标 终点下标 权重 (用空格隔开)");
for(i = 0; i < Graph->Ne; i++){
scanf("%d %d %d", &E->V1, &E->V2, &E->Weight);
InsertEdge(Graph,E);
}
}
printf("输入顶点名称:");
getchar(); // 读取上一个 scanf() 函数中留下的换行符
for(V = 0; V < Graph->Nv; V++){
scanf("%c", &(Graph->G[V].Data));
}
return Graph;
}
/* 深度优先遍历操作
* 函数名:DFS
* 说明:入参 (LGraph Graph, Vertex V),出参 LGraph Graph
* 测试输入:0 3 1 0 1 1 1 3 1 1 2 1 A B C D
*/
void DFS(LGraph Graph, Vertex V){
/* 以 V 为出发点对邻接表存储的图 Graph 进行 DFS 搜索 */
PtrToAdjVNode W;
Visit(Graph, V);
Visited[V] = true;
for(W = Graph->G[V].FirstEdge; W; W = W->Next){
if(!Visited[W->AdjV]){
DFS(Graph, W->AdjV);
}
}
}
```
修改的地方:
1. `scanf("%d %d %d",&E->V1;&E->V2;E->Weight);` 这行代码中,应该使用逗号分隔开每个变量,而不是分号。应该改为 `scanf("%d %d %d", &E->V1, &E->V2, &E->Weight);`。
2. `scanf("%c",&(Graph->G[V].Data);` 这行代码中,由于上一次输入时输入了一个整数,此时缓冲区中还有一个换行符,所以需要在读取字符前加一个 `getchar()` 函数读取上一个 `scanf()` 函数中留下的换行符。同时,由于每个顶点的名称只需要一个字符,所以这里直接使用 `%c` 读取即可。
3. 在 `DFS` 函数中,应该将 `Visited[V]` 标记为 true,表示已经访问过了。
阅读全文
相关推荐
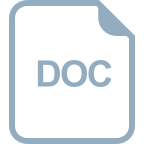
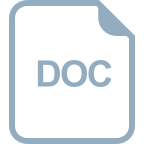
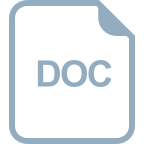













