用C语言实现编程实现,输入n个点,m条边的图,用BFS来遍历这个图
时间: 2024-03-15 11:46:30 浏览: 55
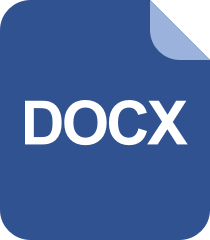
C语言:图的DFS和BFS(内附代码和算法思路).docx
好的,以下是 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_N 1000 // 图中最大节点数
// 定义一个图结构体
typedef struct Graph {
int num_nodes; // 节点数
int adjacency_matrix[MAX_N][MAX_N]; // 邻接矩阵
} Graph;
// BFS 遍历
void bfs(Graph *g, int start_node) {
bool visited[MAX_N] = {false};
int queue[MAX_N];
int front = 0, rear = 0;
queue[rear++] = start_node;
visited[start_node] = true;
while (front != rear) {
int node = queue[front++];
printf("%d ", node);
for (int neighbor = 0; neighbor < g->num_nodes; neighbor++) {
if (g->adjacency_matrix[node][neighbor] == 1 && !visited[neighbor]) {
queue[rear++] = neighbor;
visited[neighbor] = true;
}
}
}
}
int main() {
Graph g;
int n, m;
// 输入节点数和边数
scanf("%d %d", &n, &m);
g.num_nodes = n;
// 初始化邻接矩阵
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
g.adjacency_matrix[i][j] = 0;
}
}
// 输入边
for (int i = 0; i < m; i++) {
int from, to;
scanf("%d %d", &from, &to);
g.adjacency_matrix[from][to] = g.adjacency_matrix[to][from] = 1;
}
// 从节点 0 开始遍历
bfs(&g, 0);
return 0;
}
```
输入格式为:
```
节点数 边数
边1起点 边1终点
边2起点 边2终点
...
```
例如,输入:
```
5 6
0 1
0 2
1 2
1 3
2 3
2 4
```
输出结果为:
```
0 1 2 3 4
```
阅读全文
相关推荐
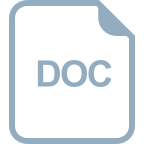
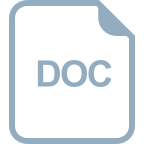


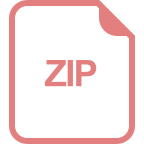
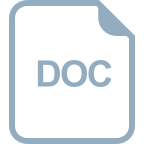










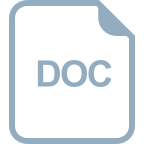
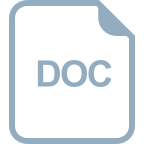