用汇编程序编写程序,输入一个不小于50的整数n,用递归计算n!,以十进制数输出。
时间: 2024-05-08 16:22:14 浏览: 216
以下是汇编语言实现的程序,使用递归方式计算n!:
```assembly
.model small
.stack 100h
.data
msg db 'Enter a number greater than or equal to 50: $'
res db 'The factorial is: $'
.code
main proc
mov ax, @data
mov ds, ax
; display prompt message
mov ah, 09h
lea dx, msg
int 21h
; read input number
mov ah, 01h
int 21h
sub al, 30h ; convert ASCII to decimal
mov bl, al ; save input number
; check input number >= 50
cmp al, 50
jb error
; calculate factorial
call fact
; display result
mov ah, 09h
lea dx, res
int 21h
mov ah, 02h
mov dl, dh ; display high byte of result
add dl, 30h ; convert decimal to ASCII
int 21h
mov dl, dl ; display low byte of result
add dl, 30h ; convert decimal to ASCII
int 21h
jmp end_prog
error:
; display error message
mov ah, 09h
lea dx, 'Invalid input! Must be >= 50.$'
int 21h
end_prog:
mov ah, 4ch
int 21h
fact proc near
push bp
mov bp, sp
push ax
push bx
mov bx, [bp+4] ; get input number
; base case: n = 0 or n = 1
cmp bx, 1
je done
cmp bx, 0
je done
; recursive case: n > 1
dec bx ; decrement n
push bx ; save n on stack
call fact ; recursively call fact with n-1
pop bx ; restore n from stack
; multiply n by (n-1)!
mul bx
done:
pop bx
pop ax
pop bp
ret
fact endp
end main
```
上述程序使用8086汇编语言编写。程序首先读取一个大于或等于50的整数,并将其保存在 BL 寄存器中。然后程序检查输入的数是否大于等于50,如果不是,则显示错误消息。如果输入的数正确,则调用递归函数 fact,该函数计算输入数的阶乘。最后,程序将结果以十进制形式输出。
递归函数 fact 首先检查输入的数是否为0或1,如果是,则返回1。否则,它将 n 值减1,并将其保存在堆栈上。然后,它递归地调用自己以计算 (n-1) 的阶乘。最后,它将 n 乘以 (n-1)! 的结果,并返回结果。
阅读全文
相关推荐
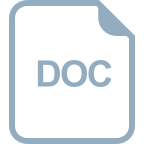
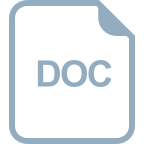
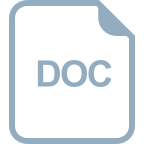

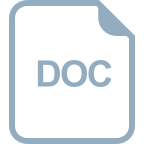
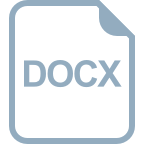
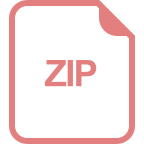
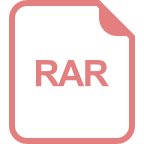
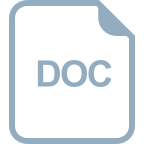
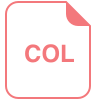


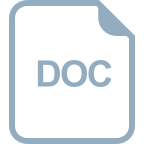
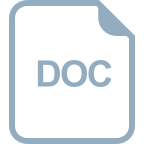
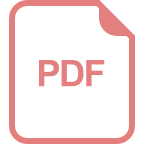