请用c++写一个程序,使用贪心算法求解最小生成树
时间: 2024-12-06 21:25:01 浏览: 11
在C++中,我们可以使用Prim's算法(一种贪心算法)来解决最小生成树问题。Prim's算法的基本思想是从一个顶点开始,每次添加一条连接当前生成树到未加入顶点中最短边的边,直到所有顶点都被包含在内。这里是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
#include <limits>
// 定义邻接矩阵表示图
class Graph {
private:
int numVertices;
std::vector<std::vector<int>> adjMatrix;
public:
Graph(int vertices) : numVertices(vertices) { adjMatrix.resize(numVertices, std::vector<int>(numVertices)); }
// 添加边并设置权重
void addEdge(int src, int dest, int weight) {
adjMatrix[src][dest] = weight;
adjMatrix[dest][src] = weight; // 对称图,不需要再次添加
}
// Prim's algorithm
std::vector<int> primMST() {
bool marked[numVertices]; // 标记数组,初始化为false
std::vector<int> mstSet; // 最小生成树边集合
// 将任意一个顶点标记为已访问
marked[0] = true;
for (int count = 0; count < numVertices - 1; count++) {
int minDistance = INT_MAX;
int minIndex;
// 找到与未访问节点相连并且距离最小的边
for (int v = 0; v < numVertices; v++) {
if (!marked[v] && adjMatrix[0][v] <= minDistance) {
minDistance = adjMatrix[0][v];
minIndex = v;
}
}
// 添加边到最小生成树
mstSet.push_back(minDistance);
marked[minIndex] = true;
// 更新与新顶点相连的未访问顶点的距离
for (int v = 0; v < numVertices; v++) {
if (!marked[v] && adjMatrix[minIndex][v] < adjMatrix[0][v]) {
adjMatrix[0][v] = adjMatrix[minIndex][v];
}
}
}
return mstSet;
}
};
int main() {
int V; // 图的顶点数
std::cout << "Enter number of vertices: ";
std::cin >> V;
Graph g(V);
// 这里可以按需要输入边的信息和权重...
std::vector<int> mstEdges = g.primMST();
std::cout << "Minimum spanning tree edges and weights:\n";
for (int i = 0; i < mstEdges.size(); i += 2) {
std::cout << "Edge " << i << ": (" << i / 2 << ", " << (i / 2 + 1) << ") with weight: " << mstEdges[i] << "\n";
}
return 0;
}
```
在这个程序中,我们首先创建了一个邻接矩阵`adjMatrix`来存储图的边及其权重。然后,在`primMST`函数中实现了Prim's算法的核心步骤。注意,实际应用中需要根据用户输入或其他数据源填充边的信息。
阅读全文
相关推荐
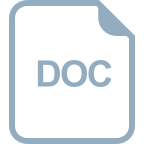
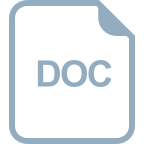
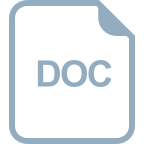








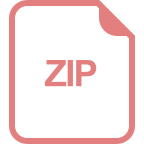
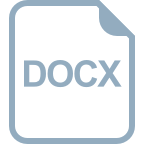
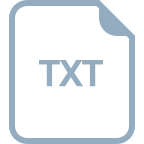
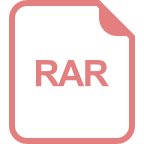



