c++ drawContours
时间: 2023-08-18 07:14:49 浏览: 50
Sure! To draw contours in C++, you can use the OpenCV library. Here's an example of how you can draw contours on an image:
```cpp
#include <opencv2/opencv.hpp>
int main()
{
// Load the image
cv::Mat image = cv::imread("path_to_image.jpg");
// Convert the image to grayscale
cv::Mat gray;
cv::cvtColor(image, gray, cv::COLOR_BGR2GRAY);
// Apply thresholding
cv::Mat thresholded;
cv::threshold(gray, thresholded, 128, 255, cv::THRESH_BINARY);
// Find contours
std::vector<std::vector<cv::Point>> contours;
cv::findContours(thresholded, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
// Draw contours on the image
cv::drawContours(image, contours, -1, cv::Scalar(0, 255, 0), 2);
// Display the result
cv::imshow("Contours", image);
cv::waitKey(0);
return 0;
}
```
Make sure to replace "path_to_image.jpg" with the actual path to your image file. This code reads an image, converts it to grayscale, applies thresholding, finds contours, and then draws them on the original image. Finally, it displays the image with the drawn contours.
Remember to install and link the OpenCV library properly in your C++ project.
相关推荐
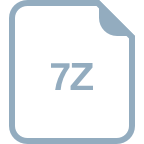
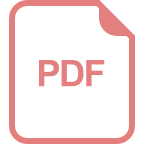
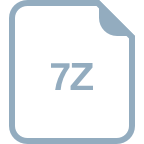














