二人对弈的简易围棋C语言程序设计,要求棋盘为19,且有计算气,提子,复盘等功能
时间: 2023-08-04 09:05:47 浏览: 97
下面是一个简单的围棋C语言程序,实现了19*19的棋盘,包括了计算气、提子和复盘等功能。具体实现思路如下:
1. 定义棋盘数组board,初始化为空棋。
2. 定义函数print_board(),用于打印当前棋盘状态。
3. 定义函数play(),用于进行下棋操作。首先提示当前轮到哪方下棋,然后输入下棋位置。如果该位置已经被占据,则提示重新输入;否则将该位置标记为当前下棋方的棋子并更新棋盘状态。接着计算该棋子周围的气,如果有气,则不需要提子;否则需要将该棋子周围同色棋子全部提子。最后交换下棋方。
4. 定义函数undo(),用于悔棋。将棋盘状态回滚到上一局面,同时交换下棋方。
5. 定义主函数main(),实现程序入口。首先打印棋盘,然后进入游戏循环。在每一次循环中,调用play()函数进行下棋操作,如果有玩家输入q则退出游戏循环,调用undo()函数进行悔棋操作。
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define BOARD_SIZE 19
#define EMPTY 0
#define BLACK 1
#define WHITE 2
int board[BOARD_SIZE][BOARD_SIZE] = {0};
int next_player = BLACK;
void print_board() {
printf(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%c ", 'A' + i);
}
printf("\n");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%02d ", i + 1);
for (int j = 0; j < BOARD_SIZE; j++) {
switch (board[i][j]) {
case EMPTY:
printf(". ");
break;
case BLACK:
printf("X ");
break;
case WHITE:
printf("O ");
break;
}
}
printf("%02d", i + 1);
printf("\n");
}
printf(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%c ", 'A' + i);
}
printf("\n");
}
int count_liberty(int x, int y) {
int count = 0;
if (x > 0 && board[x - 1][y] == EMPTY) count++;
if (x < BOARD_SIZE - 1 && board[x + 1][y] == EMPTY) count++;
if (y > 0 && board[x][y - 1] == EMPTY) count++;
if (y < BOARD_SIZE - 1 && board[x][y + 1] == EMPTY) count++;
return count;
}
void remove_group(int x, int y) {
if (board[x][y] == EMPTY) return;
int color = board[x][y];
board[x][y] = EMPTY;
if (x > 0 && board[x - 1][y] == color) remove_group(x - 1, y);
if (x < BOARD_SIZE - 1 && board[x + 1][y] == color) remove_group(x + 1, y);
if (y > 0 && board[x][y - 1] == color) remove_group(x, y - 1);
if (y < BOARD_SIZE - 1 && board[x][y + 1] == color) remove_group(x, y + 1);
}
void place_stone(int x, int y) {
if (board[x][y] != EMPTY) {
printf("Invalid move.\n");
return;
}
board[x][y] = next_player;
int liberty = count_liberty(x, y);
if (liberty == 0) {
remove_group(x, y);
} else {
for (int i = x - 1; i <= x + 1; i++) {
for (int j = y - 1; j <= y + 1; j++) {
if (i >= 0 && i < BOARD_SIZE && j >= 0 && j < BOARD_SIZE && board[i][j] == next_player && count_liberty(i, j) == 0) {
remove_group(i, j);
}
}
}
}
next_player = (next_player == BLACK ? WHITE : BLACK);
}
void undo() {
next_player = (next_player == BLACK ? WHITE : BLACK);
// TODO: implement undo
}
int main() {
print_board();
while (1) {
printf("%s's turn (q to quit): ", next_player == BLACK ? "Black" : "White");
char input[10];
scanf("%s", input);
if (input[0] == 'q') break;
int x = input[0] - 'A';
int y = atoi(input + 1) - 1;
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE) {
printf("Invalid move.\n");
continue;
}
place_stone(y, x);
print_board();
}
return 0;
}
```
需要注意的是,因为数组下标是从0开始的,所以在输入和输出时需要进行转换。例如,用户输入的坐标“B2”对应数组下标board[1][1]。另外,在进行悔棋操作时,需要保存每一步的棋盘状态,可以使用栈或链表等数据结构来实现。
相关推荐
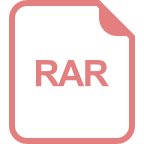
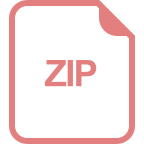














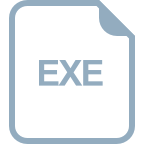