python评论词频统计
时间: 2023-06-28 07:05:29 浏览: 55
以下是Python代码实现评论词频统计的过程:
```python
import re
from collections import Counter
# 读取评论文件
with open('comments.txt', 'r', encoding='utf-8') as f:
comments = f.read()
# 将评论中的标点符号和特殊字符去除
comments = re.sub(r'[^\w\s]','',comments)
# 将评论转换为小写字母
comments = comments.lower()
# 将评论拆分为单词列表
words = comments.split()
# 统计每个单词出现的次数
word_counts = Counter(words)
# 输出前10个最常见的单词及它们的出现次数
for word, count in word_counts.most_common(10):
print(word, count)
```
其中,`comments.txt` 是包含评论内容的文本文件。首先读取评论文件,然后使用正则表达式去除标点符号和特殊字符,再将评论转换为小写字母。接着,将评论拆分为单词列表,并使用 `Counter` 对每个单词进行计数。最后,输出前 10 个最常见的单词及它们的出现次数。
相关问题
python评论词频统计结果保存为文件
要将Python评论词频统计结果保存到文件中,可以使用Python内置的文件操作函数。以下是一个简单的代码示例:
```python
from collections import Counter
# 读取评论数据
with open('comments.txt', 'r') as f:
comments = f.read()
# 分词统计
words = comments.split()
word_freq = Counter(words)
# 保存到文件
with open('word_freq.txt', 'w') as f:
for word, freq in word_freq.most_common():
f.write(f'{word}: {freq}\n')
```
这个代码示例假设评论数据已经保存在一个名为 `comments.txt` 的文件中。代码首先读取评论数据,然后使用 Python 的 `split()` 函数将其分词,并使用 `Counter` 对象进行词频统计。最后,代码将统计结果保存到名为 `word_freq.txt` 的文件中。
在保存文件时,我们使用了 `most_common()` 方法来获取词频最高的单词。这个方法返回一个包含元组的列表,每个元组包含单词和对应的词频。我们使用 `f.write()` 函数将每个元组写入文件中。
python 爬虫评论词频统计
在Python中进行爬虫评论词频统计的方法如下:
1. 首先,你需要使用爬虫获取到评论数据。你可以使用Python的requests库发送HTTP请求获取网页内容,然后使用正则表达式或者BeautifulSoup库解析网页,提取评论内容。
2. 接下来,你需要对评论内容进行分词。你可以使用Python的jieba库进行中文分词,将评论内容分割成一个个词语。
3. 然后,你可以使用Python的collections库中的Counter类来统计词频。Counter类可以接受一个可迭代对象作为输入,并返回一个字典,其中包含了每个元素出现的次数。
4. 最后,你可以根据词频结果进行排序,以便找出出现频率最高的词语。你可以使用Python的sorted函数对词频字典进行排序,然后按照降序输出结果。
下面是一个示例代码:
```python
import requests
import jieba
from collections import Counter
# 发送HTTP请求获取网页内容
url = 'http://example.com/comments'
response = requests.get(url)
html = response.text
# 使用正则表达式或BeautifulSoup库解析网页,提取评论内容
comments = extract_comments(html)
# 对评论内容进行分词
words = []
for comment in comments:
words += jieba.lcut(comment)
# 统计词频
word_counts = Counter(words)
# 按照词频进行排序
sorted_word_counts = sorted(word_counts.items(), key=lambda x: x[1], reverse=True)
# 输出词频统计结果
for word, count in sorted_word_counts:
print(word, count)
```
相关推荐
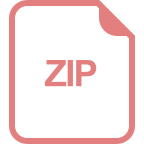
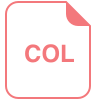
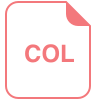
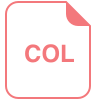
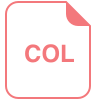
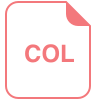







