ros一个节点创建两个ros多线程的C++程序
时间: 2024-06-10 21:10:15 浏览: 158
这里提供一个简单的例子,使用C++编写,使用ros::AsyncSpinner实现多线程:
```c++
#include <ros/ros.h>
#include <std_msgs/Int32.h>
#include <thread>
void thread1_func()
{
ros::NodeHandle nh;
ros::Publisher pub = nh.advertise<std_msgs::Int32>("thread1_topic", 10);
ros::Rate rate(10);
int count = 0;
while (ros::ok())
{
std_msgs::Int32 msg;
msg.data = count++;
pub.publish(msg);
rate.sleep();
}
}
void thread2_func()
{
ros::NodeHandle nh;
ros::Publisher pub = nh.advertise<std_msgs::Int32>("thread2_topic", 10);
ros::Rate rate(10);
int count = 0;
while (ros::ok())
{
std_msgs::Int32 msg;
msg.data = count++;
pub.publish(msg);
rate.sleep();
}
}
int main(int argc, char** argv)
{
ros::init(argc, argv, "multi_thread_node");
std::thread thread1(thread1_func);
std::thread thread2(thread2_func);
ros::AsyncSpinner spinner(2);
spinner.start();
ros::waitForShutdown();
thread1.join();
thread2.join();
return 0;
}
```
上述程序创建了两个线程,分别发布“thread1_topic”和“thread2_topic”的Int32消息。在主线程中使用ros::AsyncSpinner启动两个线程,等待ros节点关闭后,再join两个线程。
阅读全文
相关推荐
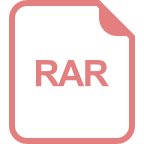

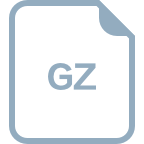










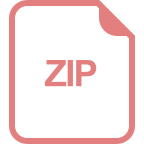
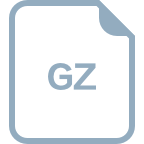
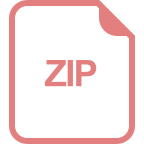
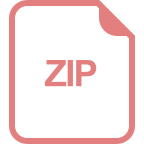