ros::spin()前面的程序加到单独的ros多线程中的C++程序
时间: 2024-05-02 14:18:55 浏览: 119
可以通过以下方式将ros::spin()前面的程序加到单独的ROS多线程中的C程序:
1. 创建一个ROS节点,命名为“my_node”。
```
ros::init(argc, argv, "my_node");
ros::NodeHandle nh;
```
2. 创建一个ROS多线程管理器,使用ROS的内置多线程库。
```
ros::AsyncSpinner spinner(1);
spinner.start();
```
3. 创建一个新的线程,在该线程中运行ROS节点。
```
pthread_t thread;
pthread_create(&thread, NULL, my_node_thread, NULL);
```
4. 在my_node_thread中定义ROS节点的回调函数和主循环。
```
void* my_node_thread(void* arg)
{
ros::NodeHandle nh;
// 定义ROS节点的回调函数
ros::Subscriber sub = nh.subscribe("topic", 10, callback);
// 定义ROS节点的主循环
while (ros::ok())
{
// 在ROS多线程管理器中处理ROS节点的回调函数
ros::spinOnce();
// 执行其他程序代码
// ...
}
return NULL;
}
```
5. 在主函数中等待线程结束。
```
pthread_join(thread, NULL);
```
完整的C程序示例:
```
#include <ros/ros.h>
#include <pthread.h>
void* my_node_thread(void* arg)
{
ros::NodeHandle nh;
// 定义ROS节点的回调函数
ros::Subscriber sub = nh.subscribe("topic", 10, callback);
// 定义ROS节点的主循环
while (ros::ok())
{
// 在ROS多线程管理器中处理ROS节点的回调函数
ros::spinOnce();
// 执行其他程序代码
// ...
}
return NULL;
}
int main(int argc, char** argv)
{
// 初始化ROS节点
ros::init(argc, argv, "my_node");
ros::NodeHandle nh;
// 创建ROS多线程管理器
ros::AsyncSpinner spinner(1);
spinner.start();
// 创建新线程,在该线程中运行ROS节点
pthread_t thread;
pthread_create(&thread, NULL, my_node_thread, NULL);
// 等待线程结束
pthread_join(thread, NULL);
return 0;
}
```
阅读全文
相关推荐






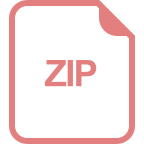
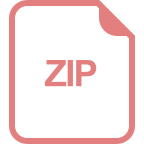
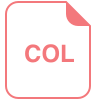
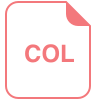
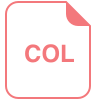
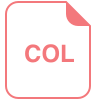
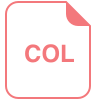
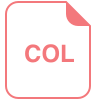
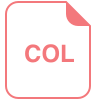
