用python实现精确线搜索+最速下降方法 求解以下二次规划问题 min f(x) =1/2x1^2+x2^2-x1x2-x1
时间: 2024-10-09 18:16:17 浏览: 56
为了用Python实现针对给定二次规划问题`min f(x) = 1/2*x1^2 + x2^2 - x1*x2 - x1`的精确线搜索和最速下降法,你可以按照以下步骤进行:
首先,定义函数`f`和它的导数`df`(梯度):
```python
import numpy as np
def objective_function(x):
x1, x2 = x
return 0.5 * x1**2 + x2**2 - x1*x2 - x1
def gradient_function(x):
x1, x2 = x
return np.array([x1 - x2 - 1, x2 - x1])
```
接着,编写精确线搜索的牛顿线性化部分和步长搜索:
```python
def line_search(f, gradient, current_x, initial_stepsize=1e-4, max_iterations=50, tolerance=1e-6):
df_dx = gradient(current_x)
alpha = initial_stepsize
for i in range(max_iterations):
candidate_x = current_x - alpha * df_dx
if f(candidate_x) <= f(current_x) and np.linalg.norm(candidate_x - current_x) < tolerance:
break
curvature = np.dot(df_dx.T, df_dx)
alpha *= -1 / curvature
else:
print("Failed to find a suitable step size after", max_iterations, "iterations.")
return alpha, candidate_x
# 更新点函数
def update_x(gradient_func, current_x, alpha):
return current_x - alpha * gradient_func(current_x)
def quasi_newton_descent(objective_function, initial_guess, max_iterations=100):
x = initial_guess
for _ in range(max_iterations):
gradient = gradient_function(x)
alpha, next_x = line_search(objective_function, gradient, x)
x = update_x(gradient_function, x, alpha)
return x
```
现在你可以调用`quasi_newton_descent`函数,传入初始猜测值,得到优化结果:
```python
initial_guess = np.array([0.0, 0.0]) # 或者根据需要设置其他初始值
optimized_solution = quasi_newton_descent(objective_function, initial_guess)
print(f"Optimized solution: {optimized_solution}")
```
阅读全文
相关推荐
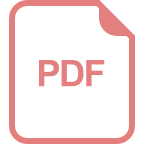
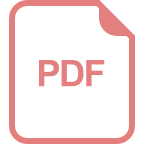
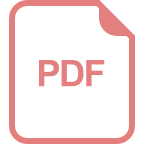















