首页arduino外部中断程序怎么写
arduino外部中断程序怎么写
时间: 2024-09-06 13:05:59 浏览: 23
在Arduino Uno(包括Mega2560)上编写外部中断程序,可以使用`attachInterrupt()`函数来配置中断。以下是基本步骤[^1]:
1. **初始化中断源**:
```cpp
// 假设你想监听pin 2的下降沿中断
attachInterrupt(digitalPinToInterrupt(2), yourInterruptHandler, FALLING);
```
这里`yourInterruptHandler`是你自定义的中断处理函数。
2. **编写中断处理函数**:
```cpp
void yourInterruptHandler() {
// 当pin 2的电平从高变低时,这个函数会被调用
Serial.println("External interrupt occurred on pin 2");
// 执行你需要的任务
}
```
对于ESP Arduino(如ESP32),外部中断的设置可能略有不同[^2],但通常也涉及到选择中断引脚并配置中断回调。具体操作可能依赖于库或框架,例如`ESP32 Interrupt Library`。
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
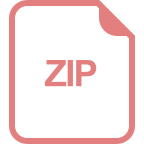
java-ssm+vue旅游资源网站实现源码(项目源码-说明文档)
旅游资源网站的主要使用者分为管理员和用户,实现功能包括管理员:首页、个人中心、用户管理、景点信息管理、购票信息管理、酒店信息管理、客房类型管理、客房信息管理、客房预订管理、交流论坛、系统管理,用户:首页、个人中心、购票信息管理、客房预订管理、我的收藏管理,前台首页;首页、景点信息、酒店信息、客房信息、交流论坛、红色文化、个人中心、后台管理、客服等功能。
项目关键技术
开发工具:IDEA 、Eclipse
编程语言: Java
数据库: MySQL5.7+
后端技术:ssm
前端技术:Vue
关键技术:springboot、SSM、vue、MYSQL、MAVEN
数据库工具:Navicat、SQLyog
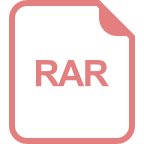
【高创新】基于粒子群优化算法PSO-Transformer-BiLSTM实现故障识别Matlab实现.rar
1.版本:matlab2014/2019a/2024a
2.附赠案例数据可直接运行matlab程序。
3.代码特点:参数化编程、参数可方便更改、代码编程思路清晰、注释明细。
4.适用对象:计算机,电子信息工程、数学等专业的大学生课程设计、期末大作业和毕业设计。
替换数据可以直接使用,注释清楚,适合新手
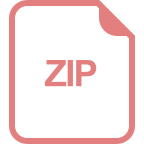
这里收集那些神奇的产品经理为我们带来的意想不到的产品功能和改版,又称_MDZZ_PM_awesome-pm.zip
这里收集那些神奇的产品经理为我们带来的意想不到的产品功能和改版,又称_MDZZ_PM_awesome-pm
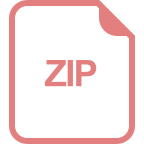
AI City track 5数据集-voc-xml格式
有戴头盔的人、未戴头盔的人、摩托车三种类别,包含736张图像、对应voc格式标签(xml)
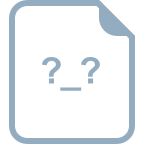
4-3_Business_BLUE_2017_16-CL-20180524MTAX.potx
微软演示材料
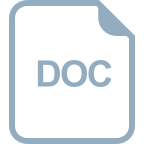
WebLogic集群配置与管理实战指南
"Weblogic 集群管理涵盖了WebLogic服务器的配置、管理和监控,包括Adminserver、proxyserver、server1和server2等组件的启动与停止,以及Web发布、JDBC数据源配置等内容。"
在WebLogic服务器管理中,一个核心概念是“域”,它是一个逻辑单元,包含了所有需要一起管理的WebLogic实例和服务。域内有两类服务器:管理服务器(Adminserver)和受管服务器。管理服务器负责整个域的配置和监控,而受管服务器则执行实际的应用服务。要访问和管理这些服务器,可以使用WebLogic管理控制台,这是一个基于Web的界面,用于查看和修改运行时对象和配置对象。
启动WebLogic服务器时,可能遇到错误消息,需要根据提示进行解决。管理服务器可以通过Start菜单、Windows服务或者命令行启动。受管服务器的加入、启动和停止也有相应的步骤,包括从命令行通过脚本操作或在管理控制台中进行。对于跨机器的管理操作,需要考虑网络配置和权限设置。
在配置WebLogic服务器和集群时,首先要理解管理服务器的角色,它可以是配置服务器或监视服务器。动态配置允许在运行时添加和移除服务器,集群配置则涉及到服务器的负载均衡和故障转移策略。新建域的过程涉及多个配置任务,如服务器和集群的设置。
监控WebLogic域是确保服务稳定的关键。可以监控服务器状态、性能指标、集群数据、安全性、JMS、JTA等。此外,还能对JDBC连接池进行性能监控,确保数据库连接的高效使用。
日志管理是排查问题的重要工具。WebLogic提供日志子系统,包括不同级别的日志文件、启动日志、客户端日志等。消息的严重级别和调试功能有助于定位问题,而日志过滤器则能定制查看特定信息。
应用分发是WebLogic集群中的重要环节,支持动态分发以适应变化的需求。可以启用或禁用自动分发,动态卸载或重新分发应用,以满足灵活性和可用性的要求。
最后,配置WebLogic的Web组件涉及HTTP参数、监听端口以及Web应用的部署。这些设置直接影响到Web服务的性能和可用性。
WebLogic集群管理是一门涉及广泛的技术学科,涵盖服务器管理、集群配置、监控、日志管理和应用分发等多个方面,对于构建和维护高性能的企业级应用环境至关重要。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
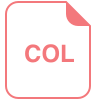
Python列表操作大全:你不能错过的10大关键技巧

# 1. Python列表基础介绍
Python列表是Python中最基本的数据结构之一,它是一个可变的序列类型,可以容纳各种数据类型,如整数、浮点数、字符串、甚至其他列表等。列表用方括号`[]`定义,元素之间用逗号分隔。例如:
```python
fruits = ["apple", "banana", "cherry"]
```
列表提供了丰富的操作方法,通过索引可以访问列表中的

编写完整java程序计算"龟兔赛跑"的结果,龟兔赛跑的起点到终点的距离为800米,乌龟的速度为1米/1000毫秒,兔子的速度为1.2米/1000毫秒,等兔子跑到第600米时选择休息120000毫秒,请编写多线程程序计算龟兔赛跑的结果。
```java
public class TortoiseAndHareRace {
private static final int TOTAL_DISTANCE = 800;
private static final int TORTOISE_SPEED = 1 * 1000; // 1米/1000毫秒
private static final int RABBIT_SPEED = 1.2 * 1000; // 1.2米/1000毫秒
private static final int REST_TIME = 120000; // 兔子休息时间(毫秒)
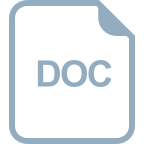
AIX5.3上安装Weblogic 9.2详细步骤
“Weblogic+AIX5.3安装教程”
在AIX 5.3操作系统上安装WebLogic Server是一项关键的任务,因为WebLogic是Oracle提供的一个强大且广泛使用的Java应用服务器,用于部署和管理企业级服务。这个过程对于初学者尤其有帮助,因为它详细介绍了每个步骤。以下是安装WebLogic Server 9.2中文版与AIX 5.3系统配合使用的详细步骤:
1. **硬件要求**:
硬件配置应满足WebLogic Server的基本需求,例如至少44p170aix5.3的处理器和足够的内存。
2. **软件下载**:
- **JRE**:首先需要安装Java运行环境,可以从IBM开发者网站下载适用于AIX 5.3的JRE,链接为http://www.ibm.com/developerworks/java/jdk/aix/service.html。
- **WebLogic Server**:下载WebLogic Server 9.2中文版,可从Bea(现已被Oracle收购)的官方网站获取,如http://commerce.bea.com/showallversions.jsp?family=WLSCH。
3. **安装JDK**:
- 首先,解压并安装JDK。在AIX上,通常将JRE安装在`/usr/`目录下,例如 `/usr/java14`, `/usr/java5`, 或 `/usr/java5_64`。
- 安装完成后,更新`/etc/environment`文件中的`PATH`变量,确保JRE可被系统识别,并执行`source /etc/environment`使更改生效。
- 在安装过程中,确保接受许可协议(设置为“yes”)。
4. **安装WebLogic Server**:
- 由于中文环境下可能出现问题,建议在英文环境中安装。设置环境变量`LANG=US`,然后运行安装命令,如:`export LANG=US; java -jar -Xmx500m server921_ccjk_generic.jar`。
- 安装路径选择`/opt`,确保在安装前有足够空间,如遇到磁盘空间不足,可以使用`chfs`命令扩展`/opt`, `/usr/`, 和 `/tmp`分区。
5. **检查和扩容磁盘空间**:
- 在开始安装前,使用`chfs -a size=XXXXM /partition_name`命令检查并扩展所需分区的大小,例如:`chfs -a size=4000M /usr`, `chfs -a size=5000M /opt`, 和 `chfs -a size=1000M /tmp`。
6. **启动设置**:
- 安装完成后,为了方便日后自动启动WebLogic Server,需要设置其开机启动。这通常涉及到修改系统服务配置文件或者使用特定工具来管理启动脚本。
7. **确认JDK版本**:
在安装JDK前,通过`java -version`命令检查已安装的JDK版本。例如,可能看到的版本信息是“Java 1.5.0”。
注意,以上步骤是基于描述中给出的版本和环境,实际操作时请根据当前的WebLogic Server和AIX版本进行适应性调整。在安装过程中,务必遵循Oracle或IBM提供的官方文档,以获取最新的安装指南和技术支持。