解释这段代码#include "interface.h" #include <stdio.h> #include <stdlib.h> #include <string.h> void inv() { invalidMessage(); failureMessage(); } make_login(U, SER, checkPass(username, passwd, &curUser)) make_login(A, DMIN, strcmp(username, ADMIN_NAME) == 0 && strcmp(passwd, ADMIN_PASS) == 0) static void USER_SignUp() { User* u = (User*)malloc(sizeof(User)); printf("Please input your username: "); scanf("%s", u->name); printf("Please input your password: "); scanf("%s", u->passwd); u->balance = 0; if (addUser(u)) successMessage(); else failureMessage(); free(u); } static HANDLER handler[] = {USER_Login, USER_SignUp, ADMIN_Login}; make_interface(M, AIN)
时间: 2024-04-19 20:23:27 浏览: 2
这段代码是一个程序的一部分,它包含了一些头文件的引用和一些函数的定义。
首先,代码中包含了"interface.h"头文件的引用,该头文件可能定义了一些接口和函数原型。
接下来,代码包含了一些标准库的引用,如<stdio.h>、<stdlib.h>和<string.h>,这些头文件提供了输入输出、内存管理和字符串处理的函数。
然后,代码定义了一个名为inv()的函数。该函数调用了invalidMessage()和failureMessage()函数,可能用于显示无效消息和失败消息。
接下来,代码定义了两个宏make_login()。这些宏可能是用来创建登录函数,并传递相应的参数。
然后,代码定义了一个名为USER_SignUp()的静态函数。该函数首先使用malloc()动态分配了一个User结构体的内存空间,然后提示用户输入用户名和密码,并将其存储在User结构体中。接下来,该函数设置了用户的余额为0,并调用了addUser()函数将用户添加到系统中。如果添加成功,则调用successMessage()函数,否则调用failureMessage()函数。最后,释放User结构体的内存空间。
最后,代码定义了一个名为handler[]的静态数组,并初始化了其中的元素。这个数组可能是用来存储处理程序的函数指针。然后使用make_interface()宏创建了一个名为M的接口。
需要注意的是,代码中出现的一些函数和宏的具体实现细节并没有给出,只是提供了它们的调用方式和可能的功能。要完全理解代码的含义,需要查看相应头文件和其他代码文件中的定义。
相关问题
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <string.h> #include <fcntl.h> #include <sys/ioctl.h> #include <linux/can.h> #include <linux/can/raw.h> #define CAN_INTERFACE "can0" // CAN接口名称 #define CAN_ID 0x123 // CAN ID int main(void) { int s; // socket描述符 struct sockaddr_can addr; struct ifreq ifr; struct can_frame frame; int nbytes; // 创建socket s = socket(PF_CAN, SOCK_RAW, CAN_RAW); if(s < 0) { perror("socket PF_CAN failed"); return 1; } // 设置CAN接口 strcpy(ifr.ifr_name, CAN_INTERFACE); ioctl(s, SIOCGIFINDEX, &ifr); addr.can_family = PF_CAN; addr.can_ifindex = ifr.ifr_ifindex; bind(s, (struct sockaddr *)&addr, sizeof(addr)); // 发送CAN数据 frame.can_id = CAN_ID; frame.can_dlc = 2; frame.data[0] = 0x01; frame.data[1] = 0x02; nbytes = write(s, &frame, sizeof(struct can_frame)); if(nbytes != sizeof(struct can_frame)) { perror("write failed"); close(s); return 1; } // 接收CAN数据 nbytes = read(s, &frame, sizeof(struct can_frame)); if(nbytes < 0) { perror("read failed"); close(s); return 1; } printf("can_id = 0x%X, can_dlc = %d, data = %02X %02X\n", frame.can_id, frame.can_dlc, frame.data[0], frame.data[1]); close(s); return 0; }这个代码gcc编译不过,重新写一下呢?
这段代码的问题在于缺少头文件的引用,需要添加以下头文件:
```c
#include <sys/types.h>
#include <sys/socket.h>
#include <net/if.h>
#include <netinet/in.h>
```
另外,建议在使用 ioctl 函数时加上错误处理,修改后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <net/if.h>
#include <netinet/in.h>
#include <linux/can.h>
#include <linux/can/raw.h>
#define CAN_INTERFACE "can0" // CAN接口名称
#define CAN_ID 0x123 // CAN ID
int main(void) {
int s; // socket描述符
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
int nbytes;
// 创建socket
s = socket(PF_CAN, SOCK_RAW, CAN_RAW);
if(s < 0) {
perror("socket PF_CAN failed");
return 1;
}
// 设置CAN接口
strcpy(ifr.ifr_name, CAN_INTERFACE);
if(ioctl(s, SIOCGIFINDEX, &ifr) < 0) {
perror("ioctl failed");
close(s);
return 1;
}
addr.can_family = PF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if(bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind failed");
close(s);
return 1;
}
// 发送CAN数据
frame.can_id = CAN_ID;
frame.can_dlc = 2;
frame.data[0] = 0x01;
frame.data[1] = 0x02;
nbytes = write(s, &frame, sizeof(struct can_frame));
if(nbytes != sizeof(struct can_frame)) {
perror("write failed");
close(s);
return 1;
}
// 接收CAN数据
nbytes = read(s, &frame, sizeof(struct can_frame));
if(nbytes < 0) {
perror("read failed");
close(s);
return 1;
}
printf("can_id = 0x%X, can_dlc = %d, data = %02X %02X\n",
frame.can_id, frame.can_dlc, frame.data[0], frame.data[1]);
close(s);
return 0;
}
```
checking whether the compiler supports GNU C++... yes checking whether g++ accepts -g... yes checking for g++ option to enable C++11 features... none needed checking dependency style of g++... gcc3 checking how to run the C preprocessor... gcc -std=gnu11 -E checking for x86_64-w64-mingw32-ranlib... no checking for ranlib... ranlib checking for x86_64-w64-mingw32-dlltool... no checking for dlltool... no checking for x86_64-w64-mingw32-ar... no checking for x86_64-w64-mingw32-lib... no checking for x86_64-w64-mingw32-link... no checking for ar... ar checking the archiver (ar) interface... ar checking dependency style of gcc -std=gnu11... gcc3 checking for x86_64-w64-mingw32-as... no checking for as... as checking whether dlltool supports --temp-prefix... yes checking whether to build a w32api package for Cygwin... no checking whether to build the Win32 libraries... yes checking whether to build the Win64 libraries... yes checking whether to build the WinARM32 libraries... no checking whether to build the WinARM64 libraries... no checking whether to use genlib... no checking whether to enable globbing... no checking whether to enable private exports... no checking whether to enable delay import libs... no checking what to provide as libmsvcrt.a... msvcrt-os checking whether to include support for Control Flow Guard... no checking whether to enable experimental features... no checking whether the compiler supports -municode... no checking for stdio.h... yes checking for stdlib.h... yes checking for string.h... yes checking for inttypes.h... yes checking for stdint.h... yes checking for strings.h... yes checking for sys/stat.h... yes checking for sys/types.h... yes checking for unistd.h... yes checking for _mingw_mac.h... no
这些都是 configure 脚本在检查编译器及相关工具是否存在和支持特定的选项和功能。其中包括:
- 检查编译器是否支持 C++11 特性,检查是否需要特定的选项来启用这些特性;
- 检查 gcc 的依赖风格;
- 检查 C 预处理器的运行方式;
- 检查是否存在 x86_64-w64-mingw32-ranlib、x86_64-w64-mingw32-dlltool、x86_64-w64-mingw32-ar、x86_64-w64-mingw32-lib、x86_64-w64-mingw32-link 和 as 等工具,如果不存在,则检查是否存在对应的 ranlib、dlltool、ar 和 as 工具;
- 检查 dlltool 是否支持 --temp-prefix 选项;
- 检查是否需要为 Cygwin 构建 w32api 包;
- 检查是否需要构建 Win32、Win64、WinARM32 和 WinARM64 库;
- 检查是否需要使用 genlib 工具;
- 检查是否需要启用 globbing、private exports 和 delay import libs 等功能;
- 检查是否支持 Control Flow Guard;
- 检查编译器是否支持 -municode 选项;
- 检查是否存在 stdio.h、stdlib.h、string.h、inttypes.h、stdint.h、strings.h、sys/stat.h、sys/types.h、unistd.h 和 _mingw_mac.h 等头文件。
这些检查是为了确保编译器和工具链的完整性和正确性,以便在进行编译和链接操作时能够顺利地进行。
相关推荐
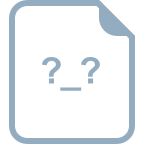
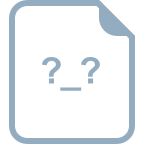
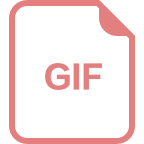













