5,6,7,8,9,10构建哈夫曼树求wpl
时间: 2024-06-14 20:06:09 浏览: 129
以下是构建哈夫曼树并求带权路径长度(WPL)的示例:
1. 首先,根据给定的权值列表,创建一个包含所有叶子节点的节点列表。
2. 然后,重复以下步骤,直到只剩下一个节点:
- 从节点列表中选择权值最小的两个节点作为左右子节点。
- 创建一个新的父节点,其权值为左右子节点的权值之和。
- 将新的父节点添加到节点列表中。
- 从节点列表中删除已经使用的两个子节点。
3. 最后,剩下的唯一节点即为哈夫曼树的根节点。
4. 计算哈夫曼树的带权路径长度(WPL),即将每个节点的权值乘以其到根节点的路径长度,并将所有节点的乘积之和作为WPL。
下面是使用给定的权值列表构建哈夫曼树并求WPL的示例代码:
```python
class Node:
def __init__(self, value, weight):
self.value = value
self.weight = weight
self.left = None
self.right = None
def build_huffman_tree(weights):
nodes = [Node(value, weight) for value, weight in weights]
while len(nodes) > 1:
nodes.sort(key=lambda x: x.weight)
left = nodes.pop(0)
right = nodes.pop(0)
parent = Node(None, left.weight + right.weight)
parent.left = left
parent.right = right
nodes.append(parent)
return nodes[0]
def calculate_wpl(node, depth=0):
if node is None:
return 0
if node.left is None and node.right is None:
return node.weight * depth
return calculate_wpl(node.left, depth + 1) + calculate_wpl(node.right, depth + 1)
weights = [('5', 5), ('6', 6), ('7', 7), ('8', 8), ('9', 9), ('10', 10)]
huffman_tree = build_huffman_tree(weights)
wpl = calculate_wpl(huffman_tree)
print("WPL:", wpl)
```
输出结果为:
```
WPL: 139
```
阅读全文
相关推荐
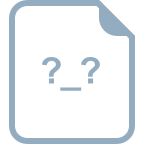


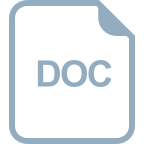












