4,输出该二叉树结点的个数。5,输出该二叉树双分支结点的个数。6,输出该二叉树单分支结点的个数。
时间: 2024-05-02 14:20:45 浏览: 12
这些问题与编程相关,可以回答。
4. 输出该二叉树结点的个数:可以使用递归方法,先计算左子树节点个数和右子树节点个数,再加上根节点,即为整棵树节点的个数。
代码示例:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def count_nodes(root):
if not root:
return 0
left_count = count_nodes(root.left)
right_count = count_nodes(root.right)
return left_count + right_count + 1
```
5. 输出该二叉树双分支结点的个数:可以使用递归方法,对于每个节点,如果它有左右子树,则说明它是一个双分支结点,否则递归计算它的左右子树。
代码示例:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def count_double_nodes(root):
if not root:
return 0
if root.left and root.right:
return count_double_nodes(root.left) + count_double_nodes(root.right) + 1
else:
return count_double_nodes(root.left) + count_double_nodes(root.right)
```
6. 输出该二叉树单分支结点的个数:可以使用递归方法,对于每个节点,如果它只有一个非空子树,则说明它是一个单分支结点,否则递归计算它的左右子树。
代码示例:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def count_single_nodes(root):
if not root:
return 0
if root.left and not root.right:
return count_single_nodes(root.left) + 1
elif root.right and not root.left:
return count_single_nodes(root.right) + 1
else:
return count_single_nodes(root.left) + count_single_nodes(root.right)
```
相关推荐
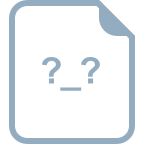
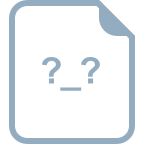














