用C语言实现定义顺序栈,实现初始化、置栈空、入栈、出栈、取栈顶元素等。然后完成数制转换
时间: 2024-10-28 10:06:48 浏览: 55
在C语言中,我们可以使用数组来实现一个简单的顺序栈。首先,定义一个结构体来存储整数值,并包含一个数组用于存放数据,以及两个指针top作为栈顶和bottom作为底端。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Stack {
int* data;
int capacity;
int top;
} Stack;
// 初始化栈
Stack* create_stack(int size) {
Stack* stack = (Stack*)malloc(sizeof(Stack));
if (!stack) {
printf("Memory allocation failed.\n");
return NULL;
}
stack->data = (int*)malloc(size * sizeof(int));
stack-1;
return stack;
}
// 置栈为空
void empty_stack(Stack* stack) {
stack->top = -1;
}
// 入栈
void push(Stack* stack, int value) {
if (stack->top == stack->capacity - 1) {
printf("Stack is full.\n");
return;
}
stack->data[++stack->top] = value;
}
// 出栈
int pop(Stack* stack) {
if (stack->top == -1) {
printf("Stack is empty.\n");
return -1; // 返回特殊值表示栈空
}
return stack->data[stack->top--];
}
// 取栈顶元素
int peek(Stack* stack) {
if (stack->top == -1) {
printf("Stack is empty.\n");
return -1; // 返回特殊值表示栈空
}
return stack->data[stack->top];
}
// 数制转换函数示例,这里仅展示十进制转二进制的基本思路
int decimal_to_binary(int num) {
int binary[32]; // 假设最大支持32位整数
int i = 0;
while (num > 0) {
binary[i++] = num % 2;
num /= 2;
}
for (int j = i - 1; j >= 0; j--) {
printf("%d", binary[j]);
}
return 0;
}
// 示例
int main() {
Stack* s = create_stack(5); // 创建一个容量为5的栈
push(s, 10);
push(s, 20);
printf("Top element is: %d\n", peek(s)); // 输出:Top element is: 20
printf("Stack after popping: %d\n", pop(s)); // 输出:Stack after popping: 10
empty_stack(s); // 空栈
free(s->data);
free(s);
return 0;
}
```
阅读全文
相关推荐
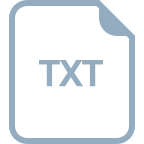
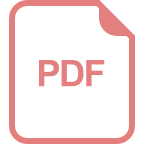
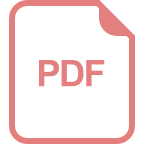


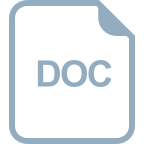
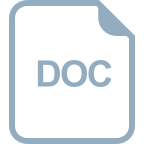


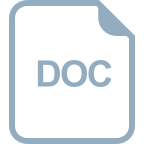








