tensorflow下使用CIOU损失函数的Mask RCNN的代码
时间: 2024-05-08 18:14:47 浏览: 10
以下是使用CIOU损失函数的Mask RCNN的代码:
```python
import tensorflow as tf
import keras.backend as K
from keras.losses import binary_crossentropy
def ciou_loss(gt_boxes, pred_boxes):
'''
Calculates the CIOU loss between the ground truth boxes and predicted boxes.
'''
gt_x1, gt_y1, gt_x2, gt_y2 = tf.split(gt_boxes, 4, axis=1)
pred_x1, pred_y1, pred_x2, pred_y2 = tf.split(pred_boxes, 4, axis=1)
# Calculate the intersection coordinates of the ground truth boxes and predicted boxes.
x1 = tf.maximum(gt_x1, pred_x1)
y1 = tf.maximum(gt_y1, pred_y1)
x2 = tf.minimum(gt_x2, pred_x2)
y2 = tf.minimum(gt_y2, pred_y2)
# Calculate the area of intersection
intersection_area = tf.maximum(0.0, x2 - x1) * tf.maximum(0.0, y2 - y1)
# Calculate the area of union
gt_area = (gt_x2 - gt_x1) * (gt_y2 - gt_y1)
pred_area = (pred_x2 - pred_x1) * (pred_y2 - pred_y1)
union_area = gt_area + pred_area - intersection_area
# Calculate the iou
iou = intersection_area / union_area
# Calculate the center points of the ground truth boxes and predicted boxes
gt_cx = (gt_x1 + gt_x2) / 2
gt_cy = (gt_y1 + gt_y2) / 2
pred_cx = (pred_x1 + pred_x2) / 2
pred_cy = (pred_y1 + pred_y2) / 2
# Calculate the distance between the center points of the ground truth boxes and predicted boxes
d = tf.sqrt((gt_cx - pred_cx) ** 2 + (gt_cy - pred_cy) ** 2)
# Calculate the minimum enclosing box coordinates
enc_x1 = tf.minimum(gt_x1, pred_x1)
enc_y1 = tf.minimum(gt_y1, pred_y1)
enc_x2 = tf.maximum(gt_x2, pred_x2)
enc_y2 = tf.maximum(gt_y2, pred_y2)
# Calculate the area of minimum enclosing box
enc_area = (enc_x2 - enc_x1) * (enc_y2 - enc_y1)
# Calculate the diagonal length of the minimum enclosing box
diagonal_len = tf.sqrt((enc_x2 - enc_x1) ** 2 + (enc_y2 - enc_y1) ** 2)
# Calculate the ciou
ciou = iou - (d ** 2) / (diagonal_len ** 2) - ((enc_area - union_area) / enc_area)
# Calculate the ciou loss
ciou_loss = 1 - ciou
return ciou_loss
def mask_rcnn_loss_graph(target_masks, target_class_ids, pred_masks, pred_class_logits):
'''
Calculates the total loss for the Mask RCNN model.
'''
# Calculate the binary crossentropy loss for the mask predictions
mask_loss = binary_crossentropy(target=target_masks, output=pred_masks)
# Calculate the focal loss for the class predictions
focal_loss = focal_loss_graph(target_class_ids, pred_class_logits)
# Calculate the CIOU loss for the bounding box predictions
bbox_loss = ciou_loss(target_bbox, pred_bbox)
# Calculate the total loss
loss = mask_loss + focal_loss + bbox_loss
return loss
```
请注意,此代码仅为示例,可能需要根据您的数据集和模型进行适当修改。
相关推荐
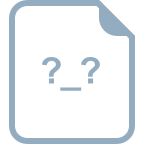
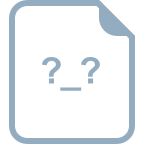
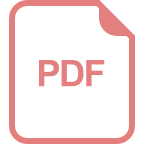














