给定一个整数n(10<n<100),求从1到n这n个整数的十进制表示中1出现的次数,用C语言写代码举例
时间: 2024-08-14 16:09:05 浏览: 44
在C语言中,我们可以使用位运算来计算从1到n这n个整数的二进制表示中1的总出现次数。因为每一位的二进制形式中1的数量等于该位的权重(如果该位为1,则贡献1次,0则不计)。当我们将每个整数转换成二进制并按位相加时,实际上就是累计了所有数字中的1的总数。
以下是一个简单的C函数示例,它实现了这个功能:
```c
#include <stdio.h>
int countOnes(int n) {
int count = 0;
while (n > 0) {
// 将n右移一位相当于除以2,并丢弃余数
// 通过异或操作得到1的位数,因为1 & 1 == 0,1 ^ 1 == 1
count += n & 1;
// 右移一位去掉最低位
n >>= 1;
}
return count;
}
int main() {
int n;
printf("Enter a number between 10 and 99: ");
scanf("%d", &n);
if (n >= 10 && n < 100) {
int result = countOnes(n);
printf("The number of 1s in the decimal representation from 1 to %d is %d.\n", n, result);
} else {
printf("Invalid input! Please enter a number between 10 and 99.\n");
}
return 0;
}
```
在这个例子中,`countOnes()` 函数通过位运算逐个统计1的出现次数,然后在`main()`函数中获取用户输入并验证范围,最后打印结果。
相关推荐
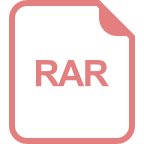
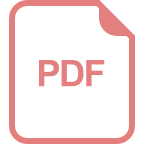
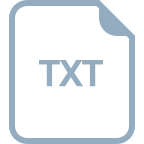














