python用分支限界法实现旅行商问题
时间: 2023-12-18 08:27:58 浏览: 80
以下是使用Python实现旅行商问题的分支限界算法的示例代码:
```python
import numpy as np
class Node:
def __init__(self, level, path, bound):
self.level = level
self.path = path
self.bound = bound
def tsp_branch_bound(cities):
n = len(cities)
# 初始化起始节点
start_node = Node(-1, [], 0)
start_node.bound = bound(start_node, cities)
# 初始化最优路径和最优距离
best_path = []
best_distance = np.inf
# 初始化队列
queue = [start_node]
while queue:
# 取出队列中的第一个节点
node = queue.pop(0)
# 如果该节点的界限小于等于当前最优距离,则不再扩展该节点
if node.bound < best_distance:
# 扩展该节点的子节点
for i in range(n):
if i not in node.path:
new_path = node.path + [i]
new_bound = bound(Node(node.level+1, new_path, 0), cities)
if new_bound < best_distance:
new_node = Node(node.level+1, new_path, new_bound)
if new_node.level == n-2:
# 如果新节点是倒数第二个节点,则直接计算最终距离
last_city = list(set(range(n)) - set(new_node.path))[0]
new_path = new_node.path + [last_city]
new_distance = distance(new_path, cities)
if new_distance < best_distance:
best_path = new_path
best_distance = new_distance
else:
# 否则将新节点加入队列
queue.append(new_node)
return best_path, best_distance
def bound(node, cities):
# 计算当前节点的界限
n = len(cities)
path = node.path
# 计算已经走过的路径长度
distance = 0
for i in range(len(path)-1):
distance += cities[path[i], path[i+1]]
# 计算当前节点到每个未走过的城市的最小距离和次小距离
min_distances = []
for i in range(n):
if i not in path:
distances = list(cities[i, j] for j in range(n) if j not in path)
min_distances.append(min(distances))
# 计算界限
bound = distance + sum(sorted(min_distances)[:2])
return bound
def distance(path, cities):
# 计算路径长度
distance = 0
for i in range(len(path)-1):
distance += cities[path[i], path[i+1]]
return distance
# 示例
cities = np.array([[0, 10, 15, 20], [10, 0, 35, 25], [15, 35, 0, 30], [20, 25, 30, 0]])
best_path, best_distance = tsp_branch_bound(cities)
print("最优路径:", best_path)
print("最优距离:", best_distance)
```
阅读全文
相关推荐
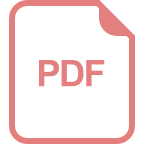




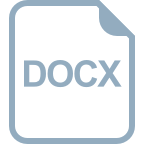
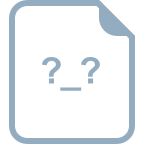
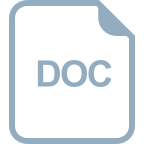







