旅行商问题 分支限界法
时间: 2023-12-18 19:28:40 浏览: 193
旅行商问题是一个NP-hard问题,因此需要采用一些高效的算法来解决。其中分支限界法是一种常用的解决TSP问题的算法。
分支限界法的基本思想是:将问题的解空间树划分为多个子树,每个子树代表一个可行解,通过限界函数对每个子树进行枝,从而减少搜索空间,提高搜索效率。具体来说,分支限界法通过不断地分支和限界,逐步缩小搜索空间,最终找到最优解。
下面是旅行商问题分支限界法的Python实现:
```python
import numpy as np
class Node:
def __init__(self, path, bound, cost):
self.path = path
self.bound = bound
self.cost = cost
def tsp_branch_bound(graph):
n = graph.shape[0]
nodes = []
for i in range(n):
path = [i]
bound = bound_func(graph, path)
cost = 0
nodes.append(Node(path, bound, cost))
nodes.sort(key=lambda x: x.bound)
best_path = None
best_cost = np.inf
while nodes:
node = nodes.pop(0)
if node.bound >= best_cost:
continue
if len(node.path) == n:
cost = node.cost + graph[node.path[-1], node.path[0]]
if cost < best_cost:
best_cost = cost
best_path = node.path + [node.path[0]]
else:
for i in range(n):
if i not in node.path:
path = node.path + [i]
bound = bound_func(graph, path)
cost = node.cost + graph[path[-2], i]
nodes.append(Node(path, bound, cost))
nodes.sort(key=lambda x: x.bound)
return best_path, best_cost
def bound_func(graph, path):
n = graph.shape[0]
bound = 0
for i in range(n):
if i not in path:
min_cost = np.min(graph[i, :])
bound += min_cost
for i in range(len(path) - 1):
bound += graph[path[i], path[i+1]]
return bound
# 示例
graph = np.array([[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]])
best_path, best_cost = tsp_branch_bound(graph)
print("最短路径为:", best_path)
print("最短路径长度为:", best_cost)
```
阅读全文
相关推荐
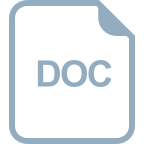
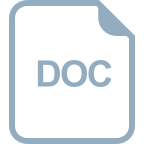
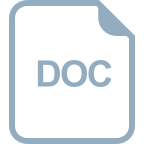

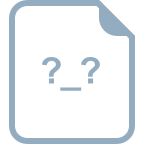







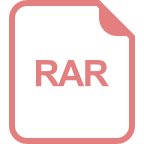





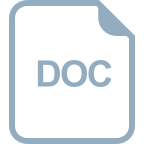