pandas 遍历文件夹下的Excel文件,并把每个Excel文件内每个sheet中,特定列包含给定值的数据,转存到同一个新的Excel中
时间: 2024-04-30 21:19:13 浏览: 16
可以使用 `pandas` 和 `os` 库实现遍历文件夹下的Excel文件,并在每个Excel文件中查找包含给定值的数据,并将结果保存到同一个新的Excel文件中。
以下是示例代码:
```python
import pandas as pd
import os
# 遍历文件夹下的Excel文件
folder_path = '/path/to/folder'
output_file = '/path/to/output/file.xlsx'
search_column = 'column_name'
search_value = 'search_value'
# 创建一个空的DataFrame来保存结果
result_df = pd.DataFrame()
for file_name in os.listdir(folder_path):
if file_name.endswith('.xlsx'):
file_path = os.path.join(folder_path, file_name)
# 遍历Excel文件中的每个sheet
for sheet_name in pd.ExcelFile(file_path).sheet_names:
# 读取当前sheet的数据
df = pd.read_excel(file_path, sheet_name=sheet_name)
# 查找包含给定值的数据
filtered_df = df[df[search_column].str.contains(search_value)]
# 如果找到了匹配的数据,将其添加到结果DataFrame中
if not filtered_df.empty:
result_df = result_df.append(filtered_df)
# 将结果保存到新的Excel文件中
result_df.to_excel(output_file, index=False)
```
在上面的代码中,我们首先指定文件夹路径 (`folder_path`)、输出文件路径 (`output_file`)、要搜索的列名 (`search_column`) 和要搜索的值 (`search_value`)。然后遍历文件夹下的所有 Excel 文件,读取每个文件中的每个 sheet,并在每个 sheet 中查找包含给定值的数据。如果找到了匹配的数据,将其添加到一个空的 DataFrame 中。最后,将结果 DataFrame 保存到输出文件中。
相关推荐
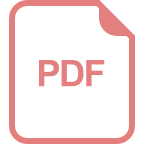
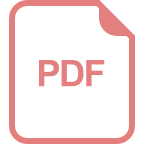
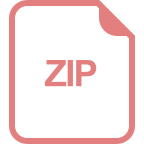
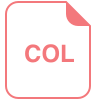
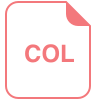
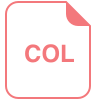
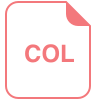
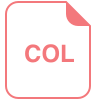









