class classify_system(QMainWindow,Ui_MainWindow): def __init__(self,parent=None): super(classify_system,self).__init__(parent) self.setupUi(self) #将输入图片按钮,pushButton与openimage关联起来 self.pushButton.clicked.connect(self.openimage) self.label_2.setAlignment(Qt.AlignCenter)#图像居中显示 def openimage(self): name,type=QFileDialog.getOpenFileName(self,"打开图片","","*.jpg;;*.png;;All Files(*)") img=cv2.imread(name) img=cv2.resize(img,(400,400),interpolation=cv2.INTER_CUBIC) qimg=qimage2ndarray.array2qimage(img) self.label_2.setPixmap(QPixmap(qimg)) self.label_2.show() img=img.astype('float')/255.0 #224*224满足模型输入要求 img=cv2.resize(img,(224,224),interpolation=cv2.INTER_CUBIC) img==np.expand_dims(img,axis=0)#添加尺寸,满足模型输入需求 MobileNet=tf.keras.applications.MobileNet(include_top=True,weights='imagenet') result=MobileNet.predict(img)#模型预测 #获得预测结果 result=tf.keras.applications.imagenet_utils.decode_predictions(result,top=1) predictClass=result[0][0][1] predictProb=result[0][0][2] print(predictClass) print(predictProb)
时间: 2024-03-11 21:51:35 浏览: 117
在你的代码中,有一个小错误,可能会导致模型的输入不正确。具体来说,这一行:
```
img==np.expand_dims(img,axis=0)#添加尺寸,满足模型输入需求
```
应该是:
```
img=np.expand_dims(img,axis=0)#添加尺寸,满足模型输入需求
```
这个错误可能是因为你想使用 `=` 赋值,但是写成了 `==`,因此导致了错误。这会导致 `img` 的值没有被更改,而是仍然是原来的值。
另外,你还需要将 `img` 转换为浮点类型,以便它在归一化时可以被正确处理。因此,你需要将这一行:
```
img=img.astype('float')/255.0
```
放在这一行后面:
```
img=cv2.resize(img,(400,400),interpolation=cv2.INTER_CUBIC)
```
这样,整个 `openimage` 函数应该像这样:
```
def openimage(self):
name,type=QFileDialog.getOpenFileName(self,"打开图片","","*.jpg;;*.png;;All Files(*)")
img=cv2.imread(name)
img=cv2.resize(img,(400,400),interpolation=cv2.INTER_CUBIC)
img=img.astype('float')/255.0
img=cv2.resize(img,(224,224),interpolation=cv2.INTER_CUBIC)
img=np.expand_dims(img,axis=0)
MobileNet=tf.keras.applications.MobileNet(include_top=True,weights='imagenet')
result=MobileNet.predict(img)
result=tf.keras.applications.imagenet_utils.decode_predictions(result,top=1)
predictClass=result[0][0][1]
predictProb=result[0][0][2]
print(predictClass)
print(predictProb)
qimg=qimage2ndarray.array2qimage(img)
self.label_2.setPixmap(QPixmap(qimg))
self.label_2.show()
```
这样应该可以解决你的问题。
阅读全文
相关推荐
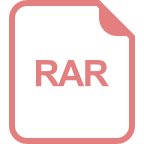
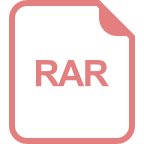
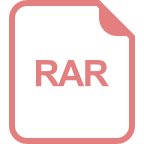
















