Write a program in C language, ask to input two positive integers, find their maximum common divisor and output
时间: 2024-05-08 08:20:14 浏览: 26
the result.
好的,您可以输入以下代码来实现您的需求:
```
#include <stdio.h>
// 定义函数,求最大公约数
int gcd(int a, int b) {
int temp;
while (b > 0) {
temp = b;
b = a % b;
a = temp;
}
return a;
}
int main() {
int a, b;
printf("请输入两个正整数:");
scanf("%d %d", &a, &b);
int result = gcd(a, b);
printf("它们的最大公约数是:%d", result);
return 0;
}
```
这个程序可以在 C 语言中运行,它会要求用户输入两个正整数,然后通过最大公约数的算法来计算它们的最大公约数并输出结果。
相关问题
Write a program to find all numbers between 'a' and 'b' (both included) such that each digit of the number is an even number, and print the results. Input There are two non-negative integers in one line: a and b Output Directly print the list
Sure, here's a Python program that will solve the problem:
```python
a, b = map(int, input().split())
# Define a function to check if a number has all even digits
def all_even_digits(n):
while n > 0:
if (n % 10) % 2 != 0:
return False
n //= 10
return True
# Loop through all numbers between a and b, check if they have all even digits, and print them if they do
for i in range(a, b+1):
if all_even_digits(i):
print(i)
```
To use this program, simply input the values of 'a' and 'b' on the first line, separated by a space. The program will then output a list of all numbers between 'a' and 'b' (inclusive) that have all even digits.
Write a program: input wo integers, calculate the difference ofsquares..
Sure, here's a Python program that takes two integers as input from the user, and calculates the difference of their squares:
```python
x = int(input("Enter the first integer: "))
y = int(input("Enter the second integer: "))
diff_of_squares = x ** 2 - y ** 2
print("The difference of squares is:", diff_of_squares)
```
In this program, we first prompt the user to enter two integers, `x` and `y`. We then calculate the difference of their squares using the formula:
- Difference of squares = x^2 - y^2
Finally, we print out the result to the user.
Note that we use the `**` operator to calculate the square of each integer. This operator raises a number to a power, so `x ** 2` means `x` raised to the power of 2 (i.e., `x` squared).
相关推荐
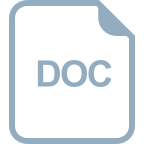













