pthread_cond_timedwait怎么用
时间: 2023-10-17 13:07:34 浏览: 96
pthread_cond_timedwait函数是一个条件变量的等待函数,可以在指定的时间内等待条件变量的状态发生变化。
它的函数原型如下:
```c
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
```
其中,
- cond:指向条件变量的指针;
- mutex:指向互斥锁的指针,在调用该函数前,必须先获得该互斥锁;
- abstime:指向结构体timespec的指针,表示等待的绝对时间。
函数的返回值为0表示成功,否则表示失败。
使用该函数需要注意以下几个问题:
1. 在调用该函数前,必须先获得互斥锁;
2. 该函数在等待期间会自动解锁互斥锁,并在等到条件变量被唤醒后再次获得互斥锁;
3. 在调用该函数后还需要检查条件变量的状态,因为该函数可能被信号打断而返回。
相关问题
pthread_cond_timedwait使用
pthread_cond_timedwait函数用于在指定的条件变量上等待,如果等待时间超过指定的超时时间,则会返回,并且可以通过返回值来判断是因为超时还是被其他线程唤醒。
函数原型如下:
```c
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
```
参数说明:
- cond:指向要等待的条件变量的指针。
- mutex:指向与条件变量相关联的互斥锁的指针。在调用pthread_cond_timedwait之前,必须先锁定互斥锁。
- abstime:指向绝对时间的指针,即等待的超时时间。
返回值:
- 若线程被唤醒并成功获取到互斥锁,则返回0。
- 若函数返回值为ETIMEDOUT,则表示等待超时。
- 其他任何非零返回值均表示出错。
使用pthread_cond_timedwait函数时,需要注意以下几点:
1. 在调用该函数之前,必须先锁定互斥锁,并且确保条件变量与互斥锁相关联。
2. 如果线程被唤醒,它会重新获取到互斥锁,从而可以继续执行后续操作。
3. abstime参数是一个绝对时间,在等待超过这个时间后,pthread_cond_timedwait将返回ETIMEDOUT错误码。
4. 注意处理返回值,可以判断等待是否超时或出错,并根据需要做相应处理。
希望以上信息能对你有所帮助!如果你有其他问题,请继续提问。
pthread_cond_timedwait 使用方法
`pthread_cond_timedwait` 函数用于在指定的时间内等待条件变量满足。以下是 `pthread_cond_timedwait` 函数的使用方法:
```c
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
```
参数说明:
- `cond`:指向条件变量的指针。
- `mutex`:指向互斥锁的指针,用于保护共享资源。
- `abstime`:指向 `struct timespec` 结构体的指针,表示等待的绝对时间。
函数返回值:
- 成功时返回 0。
- 如果等待超时,则返回 ETIMEDOUT 错误。
- 其他错误情况下返回相应的错误码。
使用 `pthread_cond_timedwait` 函数的一般步骤如下:
1. 在进入等待之前,获取互斥锁。
2. 设置等待的绝对时间。
3. 调用 `pthread_cond_timedwait` 函数,传入条件变量、互斥锁和绝对时间作为参数。
4. 根据返回值判断等待的结果,如果返回 0,则条件满足可以继续执行;如果返回 ETIMEDOUT,则表示等待超时。
5. 在条件满足或超时后,释放互斥锁。
下面是一个示例代码:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t cond = PTHREAD_COND_INITIALIZER;
void* thread_func(void* arg) {
sleep(5); // 模拟一些操作
pthread_mutex_lock(&mutex);
pthread_cond_signal(&cond); // 发送信号通知等待的线程
pthread_mutex_unlock(&mutex);
return NULL;
}
int main() {
pthread_t thread;
struct timespec timeout;
// 获取当前时间并设置等待时间为 3 秒
clock_gettime(CLOCK_REALTIME, &timeout);
timeout.tv_sec += 3;
pthread_create(&thread, NULL, thread_func, NULL);
pthread_mutex_lock(&mutex);
int result = pthread_cond_timedwait(&cond, &mutex, &timeout);
if (result == 0) {
printf("条件满足,继续执行\n");
} else if (result == ETIMEDOUT) {
printf("等待超时\n");
} else {
printf("等待出错\n");
}
pthread_mutex_unlock(&mutex);
pthread_join(thread, NULL);
return 0;
}
```
在上述示例中,我们创建了一个线程,在 5 秒后发送信号通知等待的线程。等待的线程在等待超过 3 秒后会自动返回 ETIMEDOUT 错误。
希望以上信息能够帮助到你。如果你还有其他问题,请随时提问。
阅读全文
相关推荐
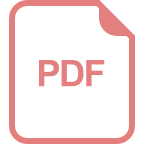
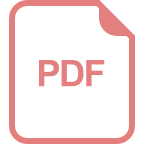
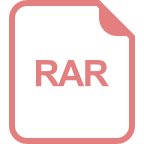













