请用c语言编写程序实现以下功能: 输入一个整数x(0<=x<=2**31-1),输出对 x执行下列操作后的结果值: 将x中从第p位开始右边的n个(二进制)位求反(即1变成0,0变成1),x的其余各位保持不变。
时间: 2024-10-19 22:07:50 浏览: 28
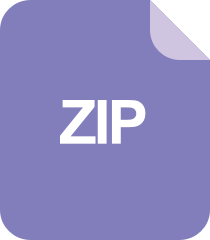
c代码-1. 功能:输入三个整数x,y,z,请把这三个数由小到大输出。

在C语言中,你可以通过以下步骤实现这个功能:
```c
#include <stdio.h>
#include <inttypes.h>
// Function to invert the specified number of bits starting from position p
uint32_t flipBits(uint32_t x, int p, int n) {
// Make sure n is within valid range and not exceeding the total number of bits in 32-bit integer
if (n < 0 || n > sizeof(x) * CHAR_BIT - p) {
printf("Invalid number of bits or position.\n");
return 0;
}
uint32_t mask = ~(~0 << n); // Create a mask with n bits set to 1 starting from position p
uint32_t shifted_mask = mask << p; // Shift the mask to start from correct bit position
// XOR the input with the shifted mask to flip the specified bits
return x ^ shifted_mask;
}
int main() {
uint32_t x;
int p, n;
printf("Enter an integer x (0 <= x <= 2^31-1): ");
scanf("%" SCNu32, &x);
printf("Enter the starting position (p) of the bit sequence: ");
scanf("%d", &p);
printf("Enter the number of bits (n) to flip: ");
scanf("%d", &n);
uint32_t result = flipBits(x, p, n);
if (result != 0) {
printf("The result after flipping bits is: %" PRIu32 "\n", result);
}
return 0;
}
```
在这个程序中,我们首先获取用户输入的整数x、位置p和需要反转的位数n。然后,我们创建了一个掩码(mask),通过异或操作来改变指定的位。如果用户输入有效,则输出翻转后的结果;否则,会提示错误。
阅读全文
相关推荐
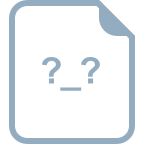















