编写一段程序,使其用管道来实现父子进程之间的进程通信。子进程向父进程发 送自己的进程标识符,以及字符串“is sending a message to parent!”。父进程则通过管道读 出子进程发来的消息,将消息显示在屏幕上,然后终止,用vs c语言
时间: 2024-11-24 17:41:53 浏览: 35
在C语言中,你可以使用`pipe()`函数创建一个管道,使得子进程能够通过该管道向父进程发送数据。以下是一个简单的示例,展示了如何使用管道实现在父子进程中进行进程间通信:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#define BUFFER_SIZE 1024
int main() {
int pipefd[2]; // 创建管道
pid_t child_pid; // 子进程PID
if (pipe(pipefd) == -1) { // 管道创建失败
perror("Pipe creation failed");
exit(1);
}
// 父进程部分
child_pid = fork(); // 创建子进程
if (child_pid < 0) { // fork失败
perror("Fork failed");
exit(1);
}
if (child_pid == 0) { // 子进程
char msg[] = "is sending a message to parent!";
write(pipefd[1], msg, strlen(msg) + 1); // 写入信息到管道
printf("Child process exiting...\n");
exit(0);
} else { // 父进程
close(pipefd[1]); // 父进程关闭写端
char buffer[BUFFER_SIZE];
read(pipefd[0], buffer, sizeof(buffer)); // 从管道读取信息
printf("Parent received from child: %s\n", buffer);
close(pipefd[0]); // 关闭读端
wait(NULL); // 等待子进程结束
printf("Parent process exiting...\n");
}
return 0;
}
```
在这个例子中,我们首先创建了一个管道,然后创建一个子进程。子进程会写入自己的标识符和消息到管道的写端,然后退出。父进程负责打开管道的读端,读取并显示接收到的信息,最后关闭管道并等待子进程结束。
阅读全文
相关推荐
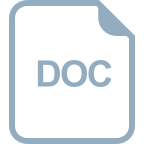
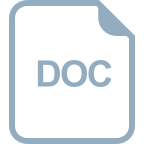
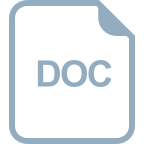















