对于任意给定的一个连通无向图的邻接矩阵,请存储为邻接表方式表示,并分别打印出无向图的邻接表。C语言
时间: 2024-12-25 13:25:46 浏览: 7
在C语言中,将一个连通无向图从邻接矩阵转换为邻接表可以提高空间效率,因为无向图中的每条边在矩阵中会被存储两次,而邻接表只需要为每个节点存储其邻居。以下是基本步骤:
首先,我们需要定义两个结构体:`Node` 表示单个节点及其连接的边,以及 `AdjacencyList` 表示邻接列表。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int value; // 节点值
struct Node* next; // 指向下一个节点的指针
} Node;
typedef struct AdjacencyList {
Node* nodes; // 链表头
} AdjacencyList;
```
然后,我们可以编写函数来处理邻接矩阵到邻接表的转换:
```c
// 将邻接矩阵转为邻接表
void matrixToAdjacencyList(int matrix[N][N], AdjacencyList** adjList, int n) {
for (int i = 0; i < n; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->value = i;
newNode->next = NULL;
for (int j = 0; j < n; j++) {
if (matrix[i][j] != 0) { // 如果有边相连
Node* existingNode = adjList[i]; // 找到节点i的链表头
while (existingNode->next != NULL) {
existingNode = existingNode->next;
}
existingNode->next = newNode; // 添加新节点到链表末尾
}
}
*adjList[i] = newNode; // 更新链表头
}
}
// 打印邻接表
void printAdjacencyList(AdjacencyList* adjList, int n) {
for (int i = 0; i < n; i++) {
printf("节点 %d 的邻接表:", i);
Node* current = adjList[i];
while (current != NULL) {
printf("(%d)->", current->value);
current = current->next;
}
printf("\n");
}
}
```
最后,你可以按照下面的方式使用这些函数:
```c
int main() {
int matrix[5][5] = {{0, 1, 0, 0, 0},
{1, 0, 1, 1, 0},
{0, 1, 0, 0, 1},
{0, 1, 0, 0, 0},
{0, 0, 1, 0, 0}};
AdjacencyList adjLists[5];
matrixToAdjacencyList(matrix, &adjLists, 5);
printAdjacencyList(adjLists, 5);
return 0;
}
```
阅读全文
相关推荐
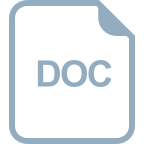















