先序和中序二叉树重构
时间: 2023-11-22 12:51:04 浏览: 75
先序遍历是指先访问根节点,然后访问左子树,最后访问右子树;中序遍历是指先访问左子树,然后访问根节点,最后访问右子树。给定一棵二叉树的先序遍历和中序遍历结果,可以重构出原二叉树。
具体步骤如下:
1. 在先序遍历中,第一个元素一定是根节点。
2. 在中序遍历中,找到根节点的位置,根节点左边的元素都是左子树的节点,右边的元素都是右子树的节点。
3. 根据中序遍历中左子树的节点个数,可以在先序遍历中找到左子树的节点,右边的节点都是右子树的节点。
4. 递归处理左子树和右子树,直到重构出整棵二叉树。
下面是一个Python实现的例子:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def buildTree(preorder, inorder):
if not preorder or not inorder:
return None
root_val = preorder[0]
root = TreeNode(root_val)
root_index = inorder.index(root_val)
left_inorder = inorder[:root_index]
right_inorder = inorder[root_index+1:]
left_preorder = preorder[1:1+len(left_inorder)]
right_preorder = preorder[1+len(left_inorder):]
root.left = buildTree(left_preorder, left_inorder)
root.right = buildTree(right_preorder, right_inorder)
return root
```
阅读全文
相关推荐
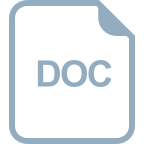
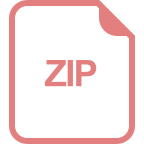
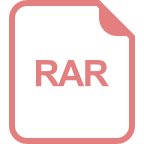
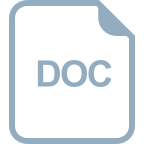


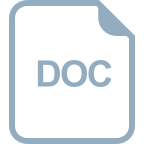








