写出蛮力法求解凸包问题的c++代码
时间: 2023-11-18 22:02:22 浏览: 36
以下是使用蛮力法求解凸包问题的C++代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Point {
int x, y;
};
// 求解向量 OA 和向量 OB 的叉积
int cross(const Point& O, const Point& A, const Point& B) {
return (A.x - O.x) * (B.y - O.y) - (A.y - O.y) * (B.x - O.x);
}
// 求解凸包问题
vector<Point> convexHull(vector<Point>& points) {
int n = points.size();
if (n <= 1) return points;
sort(points.begin(), points.end(), [](Point& a, Point& b) {
return a.x < b.x || (a.x == b.x && a.y < b.y);
});
vector<Point> hull;
for (int i = 0; i < n; ++i) {
while (hull.size() >= 2 &&
cross(hull[hull.size()-2], hull[hull.size()-1], points[i]) < 0)
hull.pop_back();
hull.push_back(points[i]);
}
// 反转向量,从右到左构建下半部分的凸包
reverse(points.begin(), points.end());
hull.pop_back();
for (int i = 0; i < n; ++i) {
while (hull.size() >= 2 &&
cross(hull[hull.size()-2], hull[hull.size()-1], points[i]) < 0)
hull.pop_back();
hull.push_back(points[i]);
}
// 删除重复的点
sort(hull.begin(), hull.end(), [](Point& a, Point& b) {
return a.x < b.x || (a.x == b.x && a.y < b.y);
});
hull.erase(unique(hull.begin(), hull.end()), hull.end());
return hull;
}
int main() {
vector<Point> points = {{0, 0}, {1, 1}, {2, 2}, {3, 3}, {3, 1}, {2, 1}};
vector<Point> hull = convexHull(points);
for (Point p : hull)
cout << "(" << p.x << ", " << p.y << ")" << endl;
return 0;
}
```
该代码的时间复杂度为 $O(n^2)$,其中 $n$ 是点集中点的数量。
相关推荐
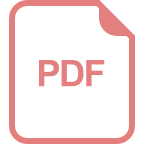














