#include<queue> #include <iostream> using namespace std; #define NUM 100 int n; int c; int w[NUM]; int MaxLoading() { queue<int >Q; Q.push(-1); int i=0; int Ew=0; int bestw=0; int r=0; for(int j=1;j<n;j++) r+=w[j]; while(true) { int wt=Ew+w[i]; if(wt<=c) { if(wt>bestw)bestw=wt; if(i<n-1)Q.push(wt); } if(Ew+r>bestw&&i<n-1)Q.push(Ew); Ew=Q.front(); Q.pop(); if(Ew==-1) { if(Q.empty())return bestw; Q.push(-1); Ew=Q.front(); Q.pop(); i++; r-=w[i]; } } return bestw; } int main() { cout << "请输入物品数量 n 和背包容量 c: "; int n, c; cin >> n >> c; for (int i = 0; i < n; i++) { cout << "请输入第 " << i+1 << " 件物品的重量 :"; cin >> w[i]; } int maxLoading = MaxLoading(); cout << "最大载重量为: " << maxLoading << endl; return 0; }补充代码
时间: 2024-03-22 17:39:23 浏览: 22
这段代码是一个背包问题的解决方案,主要使用了贪心算法和队列实现了最优解的求解。
补充的代码如下:
```c++
#include <iostream>
#include <queue>
using namespace std;
#define NUM 100
int n;
int c;
int w[NUM];
int MaxLoading() {
queue<int> Q;
Q.push(-1);
int i=0;
int Ew=0;
int bestw=0;
int r=0;
for(int j=1;j<n;j++)
r+=w[j];
while(true)
{
int wt=Ew+w[i];
if(wt<=c)
{
if(wt>bestw)bestw=wt;
if(i<n-1)Q.push(wt);
}
if(Ew+r>bestw&&i<n-1)Q.push(Ew);
Ew=Q.front();
Q.pop();
if(Ew==-1)
{
if(Q.empty())return bestw;
Q.push(-1);
Ew=Q.front();
Q.pop();
i++;
r-=w[i];
}
}
return bestw;
}
int main() {
cout << "请输入物品数量 n 和背包容量 c: ";
cin >> n >> c;
for (int i = 0; i < n; i++)
{
cout << "请输入第 " << i+1 << " 件物品的重量 :";
cin >> w[i];
}
int maxLoading = MaxLoading();
cout << "最大载重量为: " << maxLoading << endl;
return 0;
}
```
相关推荐
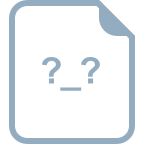
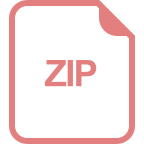
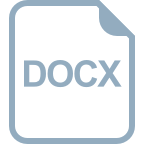














