使用系统调用pipe( )建立一个管道,然后使用系统调用fork( )创建2个子进程p1和p2。这2个子进程分别向管道中写入字符串:“Child process p1 is sending message!”和“Child process p2 is sending message!”,而父进程则从管道中读出来自两个子进程的信息,并显示在屏幕上。程序流程图
时间: 2024-02-18 14:02:51 浏览: 22
很高兴回答您的问题。下面是这个程序的流程图:
```
+------------------+
| Main Process |
+------------------+
|
| (1) create pipe
|
+---------v----------+
| |
| +-----------+ |
| | Child p1 | |
| +-----------+ |
| | (2) write to pipe
| |
| +-----------+ |
| | Child p2 | |
| +-----------+ |
| | (3) write to pipe
| |
| +-----------+ |
| | Parent | |
| +-----------+ |
| | (4) read from pipe
| |
| +-----------+ |
| | Display | |
| +-----------+ |
| |
+--------------------+
```
具体实现细节如下:
1. 在主进程中使用 `pipe()` 系统调用创建一个管道。
2. 父进程使用 `fork()` 创建子进程 p1。
3. 子进程 p1 向管道中写入字符串:“Child process p1 is sending message!”。
4. 父进程使用 `fork()` 创建子进程 p2。
5. 子进程 p2 向管道中写入字符串:“Child process p2 is sending message!”。
6. 父进程从管道中读取来自子进程 p1 的信息。
7. 父进程从管道中读取来自子进程 p2 的信息。
8. 父进程将读取到的信息显示在屏幕上。
具体代码实现可以参考下面的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/wait.h>
#define BUFFER_SIZE 256
int main() {
int fd[2];
char buffer[BUFFER_SIZE];
pid_t pid1, pid2;
/* Create a pipe */
if (pipe(fd) == -1) {
perror("pipe");
exit(EXIT_FAILURE);
}
/* Fork the first child process */
pid1 = fork();
if (pid1 == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid1 == 0) {
/* This is the first child process */
/* Close the read end of the pipe */
close(fd[0]);
/* Write to the pipe */
char *message = "Child process p1 is sending message!";
write(fd[1], message, strlen(message));
/* Close the write end of the pipe */
close(fd[1]);
/* Exit */
exit(EXIT_SUCCESS);
}
/* Fork the second child process */
pid2 = fork();
if (pid2 == -1) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid2 == 0) {
/* This is the second child process */
/* Close the read end of the pipe */
close(fd[0]);
/* Write to the pipe */
char *message = "Child process p2 is sending message!";
write(fd[1], message, strlen(message));
/* Close the write end of the pipe */
close(fd[1]);
/* Exit */
exit(EXIT_SUCCESS);
}
/* This is the parent process */
/* Close the write end of the pipe */
close(fd[1]);
/* Read from the pipe */
int bytes_read = read(fd[0], buffer, BUFFER_SIZE);
/* Null-terminate the buffer */
buffer[bytes_read] = '\0';
/* Display the message */
printf("Received from child p1: %s\n", buffer);
/* Read from the pipe */
bytes_read = read(fd[0], buffer, BUFFER_SIZE);
/* Null-terminate the buffer */
buffer[bytes_read] = '\0';
/* Display the message */
printf("Received from child p2: %s\n", buffer);
/* Close the read end of the pipe */
close(fd[0]);
/* Wait for the children to exit */
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
/* Exit */
exit(EXIT_SUCCESS);
}
```
相关推荐
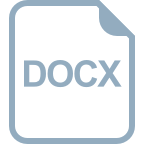
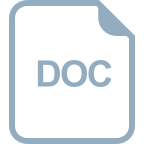
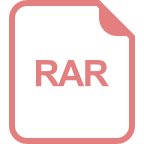













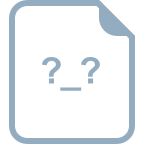
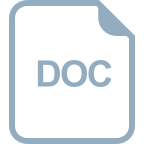
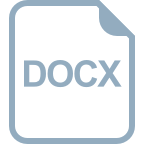