unity中通过代码实现物体部分网格顶点的删除
时间: 2023-05-22 14:02:07 浏览: 419
可以使用Mesh类中的vertices、triangles和subMeshCount属性来实现。具体步骤如下:
1. 获取需要删除的顶点的索引。
2. 遍历所有三角形,将包含需要删除的顶点的三角形的索引记录下来。
3. 根据记录的三角形索引,重新构建新的三角形数组。
4. 根据新的三角形数组,重新计算顶点数组。
5. 将新的顶点数组和三角形数组赋值给Mesh对象的vertices和triangles属性。
6. 调用Mesh对象的RecalculateNormals()方法重新计算法线。
下面是示例代码:
```csharp
Mesh mesh = GetComponent<MeshFilter>().mesh;
Vector3[] vertices = mesh.vertices;
int[] triangles = mesh.triangles;
// 获取需要删除的顶点的索引
List<int> deleteIndices = new List<int>();
for (int i = 0; i < vertices.Length; i++)
{
if (vertices[i].x < 0)
{
deleteIndices.Add(i);
}
}
// 遍历所有三角形,将包含需要删除的顶点的三角形的索引记录下来
List<int> deleteTriangles = new List<int>();
for (int i = 0; i < triangles.Length; i += 3)
{
if (deleteIndices.Contains(triangles[i]) || deleteIndices.Contains(triangles[i + 1]) || deleteIndices.Contains(triangles[i + 2]))
{
deleteTriangles.Add(i);
}
}
// 根据记录的三角形索引,重新构建新的三角形数组
int[] newTriangles = new int[triangles.Length - deleteTriangles.Count];
int index = 0;
for (int i = 0; i < triangles.Length; i += 3)
{
if (!deleteTriangles.Contains(i))
{
newTriangles[index++] = triangles[i];
newTriangles[index++] = triangles[i + 1];
newTriangles[index++] = triangles[i + 2];
}
}
// 根据新的三角形数组,重新计算顶点数组
Vector3[] newVertices = new Vector3[newTriangles.Length];
for (int i = 0; i < newTriangles.Length; i++)
{
newVertices[i] = vertices[newTriangles[i]];
newTriangles[i] = i;
}
// 将新的顶点数组和三角形数组赋值给Mesh对象的vertices和triangles属性
mesh.vertices = newVertices;
mesh.triangles = newTriangles;
// 调用Mesh对象的RecalculateNormals()方法重新计算法线
mesh.RecalculateNormals();
```
注意:这段代码只是一个示例,实际使用时需要根据具体情况进行修改和优化。
相关推荐
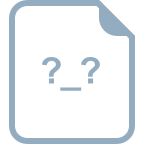
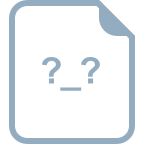
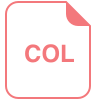
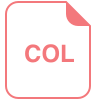
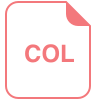
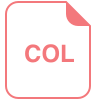










