写一个pcl将txt格式转化为pcd
时间: 2023-08-11 10:05:06 浏览: 206
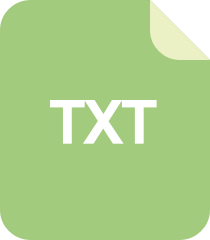
TXT转换成pcd格式的文件

以下是使用PCL库将txt格式转换为pcd格式的代码示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
void txt_to_pcd(const std::string& txt_file, const std::string& pcd_file)
{
// 读取txt文件中的点云数据
std::ifstream fin(txt_file);
if (!fin.is_open()) {
std::cerr << "无法打开文件 " << txt_file << "!" << std::endl;
return;
}
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
float x, y, z;
while (fin >> x >> y >> z) {
pcl::PointXYZ point;
point.x = x;
point.y = y;
point.z = z;
cloud->points.push_back(point);
}
fin.close();
// 保存点云数据为pcd文件
pcl::io::savePCDFileBinary(pcd_file, *cloud);
std::cout << txt_file << " 转换为 " << pcd_file << " 完成!" << std::endl;
}
void batch_txt_to_pcd(const std::string& folder_path)
{
// 创建输出文件夹
std::string pcd_folder = folder_path + "/pcd_files";
if (!std::filesystem::exists(pcd_folder)) {
std::filesystem::create_directory(pcd_folder);
}
// 遍历文件夹中的txt文件,逐个转换为pcd文件
for (const auto& entry : std::filesystem::directory_iterator(folder_path)) {
if (entry.path().extension() == ".txt") {
std::string txt_file = entry.path().string();
std::string pcd_file = pcd_folder + "/" + entry.path().stem().string() + ".pcd";
txt_to_pcd(txt_file, pcd_file);
}
}
}
// 测试
int main()
{
std::string folder_path = "/path/to/folder";
batch_txt_to_pcd(folder_path);
return 0;
}
```
将上述代码保存为C++源代码文件,然后使用支持PCL库的编译器编译并运行即可批量将文件夹中的txt文件转换为pcd文件,并保存在与txt文件相同的文件夹中的`pcd_files`子文件夹中。注意,需要将`/path/to/folder`替换为要转换的文件夹路径。
阅读全文
相关推荐
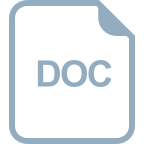
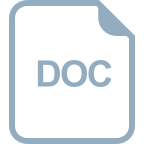

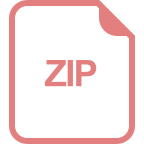
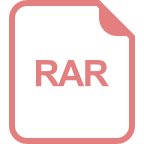




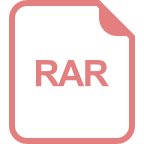







